分别用C语言和C++解决该问题
时间: 2024-09-29 14:03:18 浏览: 21
在C语言中,你可以使用指针和动态内存分配来构建这个链表,以下是基本的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int num;
int score;
struct Node* next;
} Node;
Node* create_linked_list(char* input) {
char* data = strtok(input, ",");
Node* head = (Node*)malloc(sizeof(Node));
head->num = atoi(data);
head->score = atoi(strtok(NULL, ","));
head->next = NULL;
while (data != NULL) {
data = strtok(NULL, ",");
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->num = atoi(data);
newNode->score = atoi(strtok(NULL, ","));
newNode->next = head->next;
head->next = newNode;
}
return head;
}
// 输出链表
void print_list(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("[num=%d,score=%d] -> ", temp->num, temp->score);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
char input[] = "201,98,202,94,203,89";
Node* list = create_linked_list(input);
print_list(list);
free(list); // 注意释放内存
return 0;
}
```
在C++中,可以使用标准模板库(STL)中的`std::list`来更简洁地表示链表,以下是代码示例:
```cpp
#include <iostream>
#include <sstream>
#include <vector>
struct Node {
int num;
int score;
Node* next;
};
Node* create_linked_list(std::string input) {
std::istringstream iss(input);
std::vector<std::string> tokens;
std::getline(iss, tokens[0]); // 获取头节点
Node* head = new Node{stoi(tokens[0].substr(tokens[0].find_last_of(".") + 1)), stoi(tokens[0].substr(0, tokens[0].find_last_of("."))), nullptr};
for (size_t i = 1; i < tokens.size(); i += 2) {
Node* newNode = new Node{stoi(tokens[i].substr(tokens[i].find_last_of(".") + 1)), stoi(tokens[i].substr(0, tokens[i].find_last_of(".")))};
newNode->next = head->next;
head->next = newNode;
}
return head;
}
void print_list(Node* head) {
Node* temp = head;
while (temp) {
std::cout << "[num=" << temp->num << ",score=" << temp->score << "]" << " -> ";
temp = temp->next;
}
std::cout << "NULL" << std::endl;
}
int main() {
std::string input = "201,98,202,94,203,89";
Node* list = create_linked_list(input);
print_list(list);
delete list; // 注意释放内存
return 0;
}
```
以上代码都假设了输入的字符串格式已经预处理过,只包含有效的学号和成绩。
相关推荐
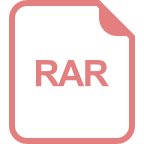
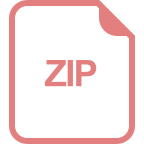
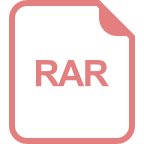














