利用java编写一个五子棋程序,程序包括Chess,Chessboard,Gamemain
时间: 2024-06-09 10:04:29 浏览: 10
以下是Java实现五子棋程序的示例代码:
1. Chess类
```java
public class Chess {
private int x;
private int y;
private int color; // 0表示黑子,1表示白子
public Chess(int x, int y, int color) {
this.x = x;
this.y = y;
this.color = color;
}
public int getX() {
return x;
}
public void setX(int x) {
this.x = x;
}
public int getY() {
return y;
}
public void setY(int y) {
this.y = y;
}
public int getColor() {
return color;
}
public void setColor(int color) {
this.color = color;
}
}
```
2. Chessboard类
```java
public class Chessboard {
private int[][] board; // 棋盘
private int size; // 棋盘大小
private int turn; // 轮到哪一方下棋,0表示黑子,1表示白子
private int count; // 已下棋子数量
private List<Chess> history; // 棋谱
public Chessboard(int size) {
this.size = size;
board = new int[size][size];
turn = 0;
count = 0;
history = new ArrayList<>();
}
public boolean canPut(int x, int y) {
return board[x][y] == 0;
}
public void putChess(int x, int y) {
Chess chess = new Chess(x, y, turn);
board[x][y] = turn + 1;
history.add(chess);
turn = 1 - turn;
count++;
}
public boolean checkWin(int x, int y) {
int color = board[x][y];
int count = 1;
int i, j;
// 横向
for (i = x - 1; i >= 0 && board[i][y] == color; i--, count++);
for (i = x + 1; i < size && board[i][y] == color; i++, count++);
if (count >= 5) {
return true;
}
// 纵向
count = 1;
for (j = y - 1; j >= 0 && board[x][j] == color; j--, count++);
for (j = y + 1; j < size && board[x][j] == color; j++, count++);
if (count >= 5) {
return true;
}
// 左上到右下
count = 1;
for (i = x - 1, j = y - 1; i >= 0 && j >= 0 && board[i][j] == color; i--, j--, count++);
for (i = x + 1, j = y + 1; i < size && j < size && board[i][j] == color; i++, j++, count++);
if (count >= 5) {
return true;
}
// 右上到左下
count = 1;
for (i = x - 1, j = y + 1; i >= 0 && j < size && board[i][j] == color; i--, j++, count++);
for (i = x + 1, j = y - 1; i < size && j >= 0 && board[i][j] == color; i++, j--, count++);
if (count >= 5) {
return true;
}
return false;
}
public boolean isFull() {
return count == size * size;
}
public int[][] getBoard() {
return board;
}
public List<Chess> getHistory() {
return history;
}
public int getTurn() {
return turn;
}
}
```
3. Gamemain类
```java
import java.util.Scanner;
public class Gamemain {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入棋盘大小:");
int size = scanner.nextInt();
Chessboard chessboard = new Chessboard(size);
while (true) {
System.out.print("请输入下棋的坐标(x,y):");
int x = scanner.nextInt();
int y = scanner.nextInt();
if (x < 0 || x >= size || y < 0 || y >= size) {
System.out.println("坐标不合法,请重新输入!");
continue;
}
if (!chessboard.canPut(x, y)) {
System.out.println("该位置已有棋子,请重新输入!");
continue;
}
chessboard.putChess(x, y);
printBoard(chessboard.getBoard());
if (chessboard.checkWin(x, y)) {
System.out.println("恭喜" + (chessboard.getTurn() == 1 ? "白方" : "黑方") + "获胜!");
break;
}
if (chessboard.isFull()) {
System.out.println("平局!");
break;
}
}
}
public static void printBoard(int[][] board) {
int size = board.length;
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
switch (board[i][j]) {
case 0:
System.out.print("● ");
break;
case 1:
System.out.print("○ ");
break;
default:
System.out.print("+ ");
break;
}
}
System.out.println();
}
}
}
```
运行程序后,输入棋盘大小,然后输入下棋的坐标就可以开始游戏了。棋盘上用黑子和白子分别表示两方,空位用加号表示。游戏结束后会显示胜利方或平局。
相关推荐
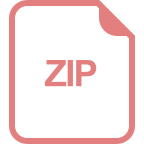
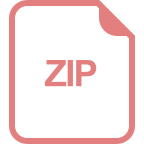














