输入一个二叉树,用一位数组存储,完成: 1、二叉树的层序输出 2、输入一个结点值,求其左右孩子 3、输入一个结点值,求其父结点 4、求树的深度
时间: 2023-06-06 09:04:20 浏览: 42
1. 二叉树的层序输出:
可以用队列实现二叉树的层序遍历,具体步骤如下:
1)将根节点入队;
2)当队列不为空时,取出队首节点并输出,将其左右孩子节点入队;
3)重复步骤2直到队列为空。
code:
```python
def level_order(root):
if not root:
return []
res = []
queue = [root]
while queue:
node = queue.pop(0)
res.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
return res
```
2. 输入一个结点值,求其左右孩子:
遍历整个树,找到目标结点,然后返回其左右孩子即可。
code:
```python
def find_node(root, target):
if not root:
return None
if root.val == target:
return root
left = find_node(root.left, target)
if left:
return left
return find_node(root.right, target)
def get_children(root, target):
node = find_node(root, target)
if node:
left_child = node.left.val if node.left else None
right_child = node.right.val if node.right else None
return left_child, right_child
return None, None
```
3. 输入一个结点值,求其父结点:
同样遍历整个树,找到目标结点的父结点即可。由于此处不是一颗二叉搜索树,需要依次比较左右子树的结点值判断当前结点是否为目标结点的父结点。
code:
```python
def find_parent(root, target):
if not root:
return None
if root.left and root.left.val == target:
return root
if root.right and root.right.val == target:
return root
left = find_parent(root.left, target)
if left:
return left
return find_parent(root.right, target)
def get_parent(root, target):
if not root or root.val == target:
return None
parent = find_parent(root, target)
if parent:
return parent.val
return None
```
4. 求树的深度:
树的深度即为根节点到最远叶子节点的距离,可以用递归实现。
code:
```python
def max_depth(root):
if not root:
return 0
left_depth = max_depth(root.left)
right_depth = max_depth(root.right)
return max(left_depth, right_depth) + 1
```
相关推荐
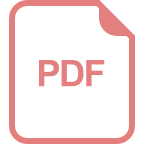
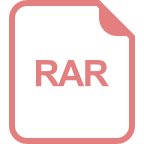
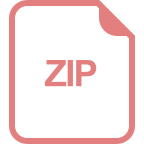















