return cv2.imdecode(np.fromfile(path, np.uint8), flags)
时间: 2023-11-13 17:02:32 浏览: 216
这是一段Python代码,使用OpenCV库中的imdecode函数将指定路径下的图像文件读入内存并解码为图像数据。具体来说,np.fromfile函数会从指定路径读取文件内容,并将其转换为一个numpy数组,数据类型为uint8。这个数组包含了图像文件的所有数据。然后,将这个numpy数组作为参数传递给imdecode函数,同时还需要指定解码标志(flags)。最后,imdecode函数会返回解码后的图像数据。
相关问题
LiTS2017数据集的路径为C:\Users\Administrator\Desktop\LiTS2017。 LiTS2017数据集里面包含Training Batch 1和Training Batch 2两个文件夹。Training Batch 1文件夹里面有图像,图像的拓展名为.nii。Training Batch 2文件夹里面有标签,标签拓展名为.nii。 请将我的LiTS2017数据集的3D图像变成2D、RGB三通道的png格式的图像。数据集的3D标签变成2D单通道的png格式标签。 并将其裁剪为单个肝脏区域。同时,移除标签中无肝脏的图片以及肝脏在图片中面积占比小于2%的切片,对数据集进行增强操作,如旋转、翻转、缩放等,以扩充数据集,提高模型的泛化能力。 保存路径为C:\Users\Administrator\Desktop\2D-LiTS2017,帮我创建保存图像与标签的文件夹,并且将转换好的数据保存到对应的文件夹。
好的,我明白了你的要求。为了实现这个任务,需要使用Python编程语言和一些常用的科学计算库,如Numpy、SimpleITK、Pillow和OpenCV。以下是代码实现的步骤:
1. 导入所需的Python库。
```python
import os
import numpy as np
import SimpleITK as sitk
from PIL import Image
import cv2
```
2. 定义一些辅助函数,用于读取、保存和处理图像。
```python
def read_image(file_name):
# 读取.nii格式的图像文件
itk_image = sitk.ReadImage(file_name)
# 将图像转换为Numpy数组
np_array = sitk.GetArrayFromImage(itk_image)
return np_array
def save_image(image, file_name):
# 将Numpy数组转换为PIL图像
pil_image = Image.fromarray(image.astype(np.uint8))
# 保存为.png格式的图像文件
pil_image.save(file_name)
def normalize_image(image):
# 将图像像素值归一化到[0, 255]的范围
min_value = np.min(image)
max_value = np.max(image)
image = (image - min_value) / (max_value - min_value) * 255
return image.astype(np.uint8)
def crop_image(image, mask):
# 根据标签的位置将图像裁剪为单个肝脏区域
x, y, w, h = cv2.boundingRect(mask)
return image[y:y+h, x:x+w], mask[y:y+h, x:x+w]
def remove_small_regions(mask, threshold=0.02):
# 移除标签中面积占比小于2%的切片
n_slices = mask.shape[0]
for i in range(n_slices):
slice_mask = mask[i]
# 计算标签面积和整个切片面积
label_area = np.sum(slice_mask == 1)
total_area = slice_mask.size
# 如果标签面积占比小于阈值,则将该切片的标签移除
if label_area / total_area < threshold:
mask[i] = 0
return mask
def augment_image(image, mask):
# 对图像进行增强操作,如旋转、翻转、缩放等
# 这里可以根据需要添加多种增强方式
angle = np.random.randint(-10, 10)
scale = np.random.uniform(0.8, 1.2)
matrix = cv2.getRotationMatrix2D((image.shape[1]/2, image.shape[0]/2), angle, scale)
image = cv2.warpAffine(image, matrix, (image.shape[1], image.shape[0]), flags=cv2.INTER_LINEAR, borderMode=cv2.BORDER_REFLECT_101)
mask = cv2.warpAffine(mask, matrix, (mask.shape[1], mask.shape[0]), flags=cv2.INTER_NEAREST, borderMode=cv2.BORDER_REFLECT_101)
return image, mask
```
3. 读取LiTS2017数据集中的图像和标签,并进行处理。
```python
# 定义LiTS2017数据集的路径
data_path = "C:/Users/Administrator/Desktop/LiTS2017"
# 定义保存转换后的图像和标签的路径
save_path = "C:/Users/Administrator/Desktop/2D-LiTS2017"
os.makedirs(save_path, exist_ok=True)
# 读取Training Batch 1文件夹中的图像
image_path = os.path.join(data_path, "Training Batch 1")
image_files = sorted(os.listdir(image_path))
images = []
for file in image_files:
if file.endswith(".nii"):
file_name = os.path.join(image_path, file)
image = read_image(file_name)
# 将3D图像变成2D、RGB三通道的png格式的图像
image = normalize_image(image)
image = cv2.merge([image]*3)
images.append(image)
# 读取Training Batch 2文件夹中的标签
label_path = os.path.join(data_path, "Training Batch 2")
label_files = sorted(os.listdir(label_path))
labels = []
for file in label_files:
if file.endswith(".nii"):
file_name = os.path.join(label_path, file)
label = read_image(file_name)
# 将3D标签变成2D单通道的png格式标签
label = (label > 0).astype(np.uint8) * 255
labels.append(label)
# 将图像和标签进行裁剪和增强操作,并保存到对应的文件夹
for i in range(len(images)):
image = images[i]
label = labels[i]
# 将图像和标签裁剪为单个肝脏区域
image, label = crop_image(image, label)
# 移除标签中无肝脏的图片以及肝脏在图片中面积占比小于2%的切片
label = remove_small_regions(label)
# 对图像进行增强操作
for j in range(5):
new_image, new_label = augment_image(image.copy(), label.copy())
# 保存图像和标签
image_file_name = os.path.join(save_path, "image_{}_{}.png".format(i, j))
label_file_name = os.path.join(save_path, "label_{}_{}.png".format(i, j))
save_image(new_image, image_file_name)
save_image(new_label, label_file_name)
```
这段代码会将LiTS2017数据集中的图像和标签转换为2D、RGB三通道的png格式的图像和2D单通道的png格式标签,并进行裁剪和增强操作,最后保存到指定的文件夹中。注意,这个任务可能需要一些时间和计算资源。
return cv2.imdecode(np.fromfile(path, np.uint8), flags) AttributeError: 'numpy.ndarray' object has no attribute 'flush'
这个错误通常是由于使用不正确的文件打开方式导致的。你可以尝试以下几种方法:
1. 确保你使用的文件打开方式正确,例如使用二进制方式打开文件。
2. 确保你的文件存在,且路径正确。
3. 尝试使用不同的文件打开方式,例如使用rb代替r。
4. 尝试使用不同的库或方法打开文件,例如使用Pillow库的Image.open()方法打开文件。
如果以上方法都不起作用,你可以提供更多的代码和错误信息,让我更好地理解问题所在并提供更准确的解决方法。
阅读全文
相关推荐
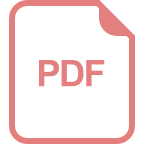
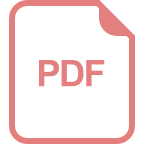
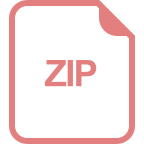
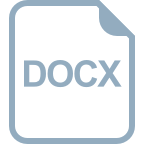
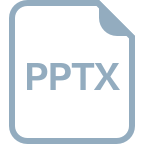
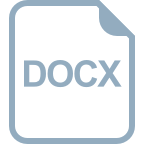
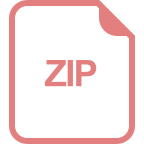
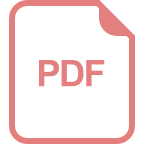
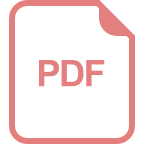
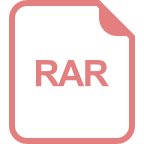
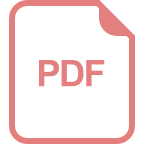
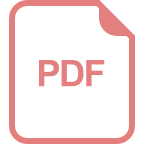
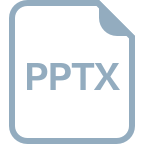
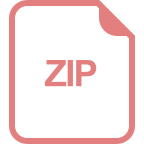
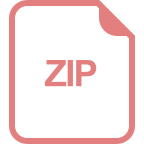