if visited(neighbor_pos(1)), neighbor_pos(2) || maze(neighbor_pos(1), neighbor_pos(2))这一行代码为什么显示错误,位置2处的索引无效,数组索引必须为正整数或逻辑值
时间: 2023-12-11 17:05:36 浏览: 69
这个错误是因为neighbor_pos返回的可能是浮点数,所以不能直接作为数组索引。你需要将neighbor_pos返回的浮点数转换为整数,才能作为索引。你可以使用floor函数或者round函数将浮点数转换为整数,例如:
```matlab
idx_1 = floor(neighbor_pos(1));
idx_2 = round(neighbor_pos(2));
if visited(idx_1, idx_2) || maze(idx_1, idx_2)
% do something
end
```
或者:
```matlab
idx_1 = round(neighbor_pos(1));
idx_2 = round(neighbor_pos(2));
if visited(idx_1, idx_2) || maze(idx_1, idx_2)
% do something
end
```
相关问题
File "D:\23101\比赛\光电赛\maze_car\测试\11111.py", line 46, in dijkstra heapq.heappush(heap, (new_distance, neighbor)) TypeError: '<' not supported between instances of 'Node' and 'Node'错误如上代码如下 def dijkstra(self, start_node): start_node.distance = 0 heap = [(start_node.distance, start_node)] while heap: (distance, node) = heapq.heappop(heap) if node.visited: continue node.visited = True for (neighbor, edge_distance) in node.neighbors: if not neighbor.visited: new_distance = node.distance + edge_distance if new_distance < neighbor.distance: neighbor.distance = new_distance heapq.heappush(heap, (new_distance, neighbor))
这个错误是由于在使用 `heapq` 堆操作时,Python 不知道如何比较节点(`Node`)对象。您需要在 `Node` 类中实现 `__lt__` 方法,以便 Python 可以比较这些对象。
例如:
```python
class Node:
def __init__(self, id):
self.id = id
self.distance = float('inf')
def __lt__(self, other):
return self.distance < other.distance
```
在这个示例中,我们将 `Node` 类的 `distance` 属性用作比较对象。请根据您的实际需要修改此代码。
# 遍历宝藏点 for treasure in treasures: while queue: current, path = queue.pop(0) if current == treasure: shortest_paths[treasure] = path break if current not in visited: visited.add(current) for neighbor in [(current[0]+1, current[1]), (current[0]-1, current[1]), (current[0], current[1]+1), (current[0], current[1]-1)]: if maze(neighbor[0], neighbor[1]) != 'wall': queue.append((neighbor, path + [current]))以上这段代码有什么功能具体怎么运行的,若去掉if maze(neighbor[0], neighbor[1]) != 'wall':会怎么样
这段代码实现了在迷宫中寻找多个宝藏点的最短路径。具体来说,代码会从起点开始,使用广度优先搜索(BFS)算法逐步扩展搜索范围,直到找到宝藏点。在搜索的过程中,代码会记录每个宝藏点的最短路径,并将其存储在字典 shortest_paths 中。
如果去掉 if maze(neighbor[0], neighbor[1]) != 'wall': 这个判断语句,代码会将所有的相邻节点都添加到队列中,包括那些被标记为墙的节点。这样会导致搜索过程变得无效,因为在这些节点中不可能存在任何路径。同时,这也会使得搜索速度变慢,因为队列中包含了大量无用的节点。
阅读全文
相关推荐
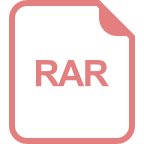
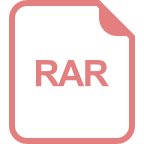
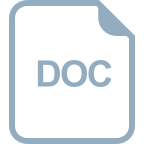
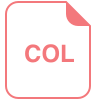









