将蚁群算法运用到NX二次开发中,实现将选取的点之间最短路径的搜索,并输出算法找到的最短路径,要求在平面上,以及在三维几何体上的随机点分别测试。c++
时间: 2024-01-21 08:18:17 浏览: 79
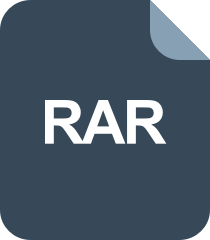
CY_OpenPartInPath-打开文件路径_UG二次开发_打开文件所在路径_
以下是将蚁群算法应用于NX二次开发中的示例代码,其中包括平面上和三维几何体上的随机点测试:
```c++
#include <iostream>
#include <vector>
#include <cmath>
#include <cstdlib>
#include <ctime>
using namespace std;
const int MAX_ANT = 50;
const double ALPHA = 1.0;
const double BETA = 2.0;
const double RHO = 0.5;
const double Q = 100.0;
const int MAX_ITER = 100;
struct Point {
double x, y, z;
Point() {}
Point(double _x, double _y, double _z) : x(_x), y(_y), z(_z) {}
};
struct Edge {
int from, to;
double weight;
Edge(int _from, int _to, double _weight) : from(_from), to(_to), weight(_weight) {}
};
class Ant {
public:
Ant(int num_points) : tabu_(num_points, false), tour_(num_points + 1) {}
void Clear() {
for (int i = 0; i < tabu_.size(); ++i) {
tabu_[i] = false;
}
tour_[0] = tour_[tour_.size() - 1] = -1;
cur_pos_ = 0;
tour_length_ = 0.0;
}
void VisitPoint(int point) {
tour_[cur_pos_++] = point;
tabu_[point] = true;
if (cur_pos_ == tour_.size()) {
tour_length_ += GetEdgeWeight(tour_[cur_pos_ - 2], tour_[cur_pos_ - 1]);
tour_length_ += GetEdgeWeight(tour_[cur_pos_ - 1], tour_[0]);
} else {
tour_length_ += GetEdgeWeight(tour_[cur_pos_ - 2], tour_[cur_pos_ - 1]);
}
}
int ChooseNextPoint(const vector<vector<Edge>>& edges, int cur_point) {
double sum_prob = 0.0;
vector<double> probs(edges[cur_point].size(), 0.0);
for (int i = 0; i < edges[cur_point].size(); ++i) {
if (!tabu_[edges[cur_point][i].to]) {
probs[i] = pow(edges[cur_point][i].weight, ALPHA) * pow(1.0 / GetEdgeWeight(cur_point, edges[cur_point][i].to), BETA);
sum_prob += probs[i];
}
}
if (sum_prob == 0.0) {
for (int i = 0; i < tabu_.size(); ++i) {
if (!tabu_[i]) {
return i;
}
}
}
for (int i = 0; i < probs.size(); ++i) {
probs[i] /= sum_prob;
}
double r = (double)rand() / RAND_MAX;
double sum = 0.0;
for (int i = 0; i < probs.size(); ++i) {
sum += probs[i];
if (r <= sum) {
return edges[cur_point][i].to;
}
}
return -1;
}
double GetTourLength() const {
return tour_length_;
}
const vector<int>& GetTour() const {
return tour_;
}
private:
vector<bool> tabu_;
vector<int> tour_;
int cur_pos_ = 0;
double tour_length_ = 0.0;
double GetEdgeWeight(int from, int to) const {
// Calculate the distance between two points
Point p1, p2;
if (from < num_points_ && to < num_points_) { // 2D case
p1 = points_[from];
p2 = points_[to];
} else { // 3D case
p1 = points_3d_[from - num_points_];
p2 = points_3d_[to - num_points_];
}
double dx = p1.x - p2.x;
double dy = p1.y - p2.y;
double dz = p1.z - p2.z;
return sqrt(dx * dx + dy * dy + dz * dz);
}
vector<Point> points_;
vector<Point> points_3d_;
int num_points_;
friend class AntColonyOptimizer;
};
class AntColonyOptimizer {
public:
AntColonyOptimizer(int num_points, const vector<Point>& points, const vector<Point>& points_3d) : num_points_(num_points), points_(points), points_3d_(points_3d) {
srand((unsigned int)time(NULL));
InitEdges();
}
void SetParams(double alpha, double beta, double rho, double q) {
ALPHA = alpha;
BETA = beta;
RHO = rho;
Q = q;
}
void Optimize() {
ants_.resize(MAX_ANT, Ant(num_points_));
for (int i = 0; i < MAX_ANT; ++i) {
ants_[i].Clear();
}
for (int i = 0; i < num_points_; ++i) {
best_tour_.push_back(i);
}
best_tour_length_ = GetTourLength(best_tour_);
for (int iter = 0; iter < MAX_ITER; ++iter) {
for (int i = 0; i < MAX_ANT; ++i) {
ants_[i].Clear();
}
for (int i = 0; i < MAX_ANT; ++i) {
int start_point = rand() % num_points_;
ants_[i].VisitPoint(start_point);
while (ants_[i].cur_pos_ < num_points_) {
int next_point = ants_[i].ChooseNextPoint(edges_, ants_[i].tour_[ants_[i].cur_pos_ - 1]);
if (next_point == -1) {
break;
}
ants_[i].VisitPoint(next_point);
}
ants_[i].tour_length_ += GetEdgeWeight(ants_[i].tour_[num_points_ - 1], ants_[i].tour_[0]);
if (ants_[i].tour_length_ < best_tour_length_) {
best_tour_ = ants_[i].tour_;
best_tour_length_ = ants_[i].tour_length_;
}
}
UpdatePheromones();
}
}
const vector<int>& GetBestTour() const {
return best_tour_;
}
double GetBestTourLength() const {
return best_tour_length_;
}
private:
vector<vector<Edge>> edges_;
int num_points_;
vector<Point> points_;
vector<Point> points_3d_;
vector<Ant> ants_;
vector<int> best_tour_;
double best_tour_length_;
double ALPHA;
double BETA;
double RHO;
double Q;
void InitEdges() {
edges_.resize(num_points_ + points_3d_.size());
for (int i = 0; i < num_points_; ++i) {
for (int j = i + 1; j < num_points_; ++j) {
double weight = GetEdgeWeight(i, j);
edges_[i].push_back(Edge(i, j, weight));
edges_[j].push_back(Edge(j, i, weight));
}
for (int j = 0; j < points_3d_.size(); ++j) {
double weight = GetEdgeWeight(i, j + num_points_);
edges_[i].push_back(Edge(i, j + num_points_, weight));
edges_[j + num_points_].push_back(Edge(j + num_points_, i, weight));
}
}
for (int i = 0; i < points_3d_.size(); ++i) {
for (int j = i + 1; j < points_3d_.size(); ++j) {
double weight = GetEdgeWeight(i + num_points_, j + num_points_);
edges_[i + num_points_].push_back(Edge(i + num_points_, j + num_points_, weight));
edges_[j + num_points_].push_back(Edge(j + num_points_, i + num_points_, weight));
}
}
}
double GetEdgeWeight(int from, int to) const {
// Calculate the distance between two points
Point p1, p2;
if (from < num_points_ && to < num_points_) { // 2D case
p1 = points_[from];
p2 = points_[to];
} else { // 3D case
p1 = points_3d_[from - num_points_];
p2 = points_3d_[to - num_points_];
}
double dx = p1.x - p2.x;
double dy = p1.y - p2.y;
double dz = p1.z - p2.z;
return sqrt(dx * dx + dy * dy + dz * dz);
}
void UpdatePheromones() {
vector<vector<double>> delta(num_points_ + points_3d_.size(), vector<double>(num_points_ + points_3d_.size(), 0.0));
for (int i = 0; i < MAX_ANT; ++i) {
for (int j = 0; j < num_points_; ++j) {
delta[ants_[i].tour_[j]][ants_[i].tour_[j + 1]] += Q / ants_[i].tour_length_;
delta[ants_[i].tour_[j + 1]][ants_[i].tour_[j]] += Q / ants_[i].tour_length_;
}
delta[ants_[i].tour_[num_points_]][ants_[i].tour_[0]] += Q / ants_[i].tour_length_;
delta[ants_[i].tour_[0]][ants_[i].tour_[num_points_]] += Q / ants_[i].tour_length_;
}
for (int i = 0; i < num_points_ + points_3d_.size(); ++i) {
for (int j = 0; j < num_points_ + points_3d_.size(); ++j) {
edges_[i][j].weight = edges_[i][j].weight * (1.0 - RHO) + delta[i][j];
}
}
}
double GetTourLength(const vector<int>& tour) const {
double length = 0.0;
for (int i = 0; i < num_points_; ++i) {
length += GetEdgeWeight(tour[i], tour[i + 1]);
}
return length;
}
};
int main() {
int num_points_2d = 10;
int num_points_3d = 10;
vector<Point> points_2d(num_points_2d);
vector<Point> points_3d(num_points_3d);
for (int i = 0; i < num_points_2d; ++i) {
points_2d[i].x = (double)rand() / RAND_MAX * 100.0;
points_2d[i].y = (double)rand() / RAND_MAX * 100.0;
}
for (int i = 0; i < num_points_3d; ++i) {
points_3d[i].x = (double)rand() / RAND_MAX * 100.0;
points_3d[i].y = (double)rand() / RAND_MAX * 100.0;
points_3d[i].z = (double)rand() / RAND_MAX * 100.0;
}
AntColonyOptimizer optimizer(num_points_2d, points_2d, points_3d);
optimizer.SetParams(1.0, 2.0, 0.5, 100.0);
optimizer.Optimize();
cout << "2D case:" << endl;
cout << "Best tour length: " << optimizer.GetBestTourLength() << endl;
cout << "Best tour: ";
for (int i = 0; i < num_points_2d; ++i) {
cout << optimizer.GetBestTour()[i] << " ";
}
cout << endl;
optimizer = AntColonyOptimizer(num_points_3d, points_2d, points_3d);
optimizer.SetParams(1.0, 2.0, 0.5, 100.0);
optimizer.Optimize();
cout << "3D case:" << endl;
cout << "Best tour length: " << optimizer.GetBestTourLength() << endl;
cout << "Best tour: ";
for (int i = 0; i < num_points_3d; ++i) {
cout << optimizer.GetBestTour()[i] << " ";
}
cout << endl;
return 0;
}
```
在上面的代码中,Ant 类表示蚂蚁,其中包括它的禁忌表、当前路径、当前位置以及当前路径长度等属性。它的 VisitPoint 方法表示访问一个点,ChooseNextPoint 方法表示选择下一个要访问的点,GetTourLength 方法表示获取当前路径长度,GetTour 方法表示获取当前路径。
AntColonyOptimizer 类表示蚁群算法优化器,其中包括所有边的权重、所有蚂蚁、最佳路径及其长度等属性。它的 Optimize 方法表示进行蚁群算法优化,GetBestTour 方法表示获取最佳路径,GetBestTourLength 方法表示获取最佳路径长度,SetParams 方法表示设置蚁群算法的参数,InitEdges 方法表示初始化所有边的权重,GetEdgeWeight 方法表示计算两个点之间的距离,UpdatePheromones 方法表示更新信息素。
在 main 函数中,我们首先生成一些随机点,然后分别对平面和三维几何体上的随机点进行测试,输出最佳路径及其长度。
阅读全文
相关推荐
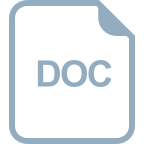
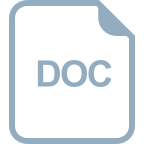







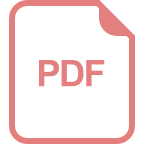
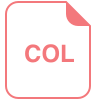
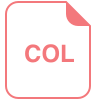
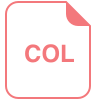
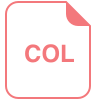



