用python代码解决八数码难题
时间: 2024-12-15 19:18:52 浏览: 15
在Python中解决八数码难题(15 Puzzle),你可以使用深度优先搜索(DFS)或广度优先搜索(BFS)等算法,配合A*搜索策略。这里给出一个简单的基于A*搜索的实现示例,假设我们已经有了一个`Puzzle`类来表示状态并计算步数以及启发函数(例如曼哈顿距离):
```python
class Puzzle:
# ... 定义状态、初始状态、移动函数等 ...
def heuristic(puzzle):
# 计算曼哈顿距离或其他启发函数
return sum(abs(i - j) for i, j in zip(puzzle.solution, puzzle.board))
def a_star_search(start, goal):
open_set = [(0, start)]
closed_set = set()
while open_set:
_, current = min(open_set, key=lambda x: x[0] + heuristic(x[1]))
if current == goal:
path = reconstruct_path(closed_set, current)
return path
open_set.remove((f_cost, current))
closed_set.add(current)
for neighbor in generate_neighbors(current):
new_f_cost = f_cost + 1
if neighbor not in closed_set and (neighbor not in open_set or new_f_cost < open_set[open_set.index(neighbor)][0]):
open_set.append((new_f_cost, neighbor))
# ... 生成邻居、重构路径等函数 ...
start_puzzle = Puzzle(initial_state)
goal_puzzle = Puzzle(goal_state)
solution_path = a_star_search(start_puzzle, goal_puzzle)
```
这个例子中,`generate_neighbors()`函数负责生成当前状态的所有合法邻居,`reconstruct_path()`则用于回溯找到最优解的路径。
阅读全文
相关推荐
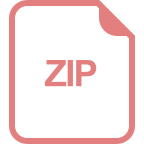
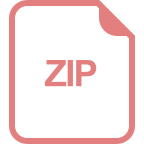



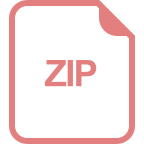
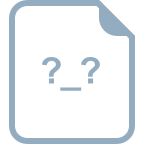







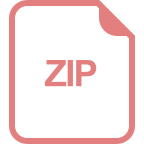
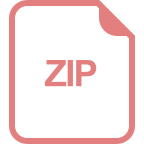