C++数据结构中给中缀算术表达式加括号代码
时间: 2024-12-06 08:21:09 浏览: 15
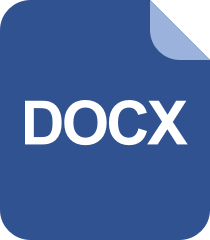
C语言:中缀算术表达式求值(栈 附答案).docx
在C++中,给中缀算术表达式添加括号通常涉及到前缀(逆波兰)或后缀(罗素)表示法,这样可以更方便地处理优先级和结合性。转换成前缀或后缀表达式后再加括号会更容易。以下是一个简单的例子,展示如何使用递归策略将中缀表达式转换为后缀(也称作RPN),并考虑了运算符的优先级:
```cpp
#include <iostream>
#include <stack>
#include <string>
// 定义操作符优先级
const char* precedence = "+-*/()";
bool isOperator(char op) {
for (int i = 0; i < sizeof(precedence); ++i) {
if (op == precedence[i])
return true;
}
return false;
}
std::string infixToPostfix(const std::string& expr) {
std::stack<char> ops;
std::string postfix;
for (char c : expr) {
if (!isOperator(c)) {
postfix += c; // 非运算符直接加入后缀
} else if (c == '(') {
ops.push(c);
} else if (c == ')') {
while (!ops.empty() && ops.top() != '(') {
postfix += ops.top();
ops.pop();
}
if (!ops.empty()) {
ops.pop(); // 弹出左括号
}
} else {
while (!ops.empty() && precedence[c] >= precedence[ops.top()]) {
postfix += ops.top();
ops.pop();
}
ops.push(c); // 运算符入栈
}
}
while (!ops.empty()) {
postfix += ops.top();
ops.pop();
}
return postfix;
}
int main() {
std::string expression = "A + B * C / D";
std::string postfix = infixToPostfix(expression);
std::cout << "Postfix expression: " << postfix << std::endl;
return 0;
}
```
在这个示例中,`infixToPostfix`函数接受一个中缀表达式,并通过栈的操作将其转换为后缀表达式。之后可以根据需要添加括号以调整运算顺序。
阅读全文
相关推荐
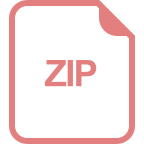
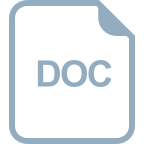
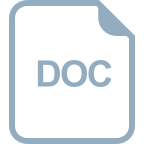
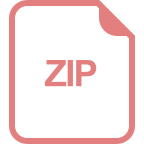











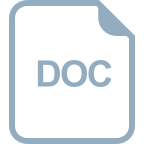
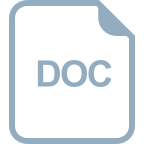