用VS写一个用二分查找法在有序表中查找关键字
时间: 2024-05-06 17:21:49 浏览: 95
好的,让我来回答你的问题。以下是使用二分查找法在有序表中查找关键字的代码:
```c++
#include <iostream>
using namespace std;
// 使用二分查找法在有序表中查找关键字
int binarySearch(int arr[], int left, int right, int key)
{
if (right >= left) {
int mid = left + (right - left) / 2;
// 如果关键字与中间元素匹配
if (arr[mid] == key)
return mid;
// 如果关键字比中间元素小,则在左子数组中查找
if (arr[mid] > key)
return binarySearch(arr, left, mid - 1, key);
// 如果关键字比中间元素大,则在右子数组中查找
return binarySearch(arr, mid + 1, right, key);
}
// 如果关键字没有出现在数组中,则返回-1
return -1;
}
int main()
{
int arr[] = { 2, 3, 4, 10, 40 };
int n = sizeof(arr) / sizeof(arr[0]);
int key = 10;
int result = binarySearch(arr, 0, n - 1, key);
(result == -1) ? cout << "Element is not present in array"
: cout << "Element is present at index " << result;
return 0;
}
```
以上代码将在有序表中查找关键字10。这个算法的时间复杂度为O(log n),因为我们在每个步骤中将搜索区域减半。
阅读全文
相关推荐




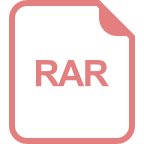










