给我python折线图,散点图,柱状图,曲面图的代码
时间: 2024-05-16 07:14:01 浏览: 96
以下是Python中使用matplotlib库绘制折线图、散点图、柱状图和曲面图的代码示例:
折线图:
```python
import matplotlib.pyplot as plt
# 数据
x = [1, 2, 3, 4, 5]
y = [4, 7, 2, 9, 3]
# 绘制折线图
plt.plot(x, y)
# 添加标题和标签
plt.title("Line Chart Example")
plt.xlabel("X Axis Label")
plt.ylabel("Y Axis Label")
# 显示图像
plt.show()
```
散点图:
```python
import matplotlib.pyplot as plt
# 数据
x = [1, 2, 3, 4, 5]
y = [4, 7, 2, 9, 3]
# 绘制散点图
plt.scatter(x, y)
# 添加标题和标签
plt.title("Scatter Plot Example")
plt.xlabel("X Axis Label")
plt.ylabel("Y Axis Label")
# 显示图像
plt.show()
```
柱状图:
```python
import matplotlib.pyplot as plt
# 数据
x = ["A", "B", "C", "D", "E"]
y = [4, 7, 2, 9, 3]
# 绘制柱状图
plt.bar(x, y)
# 添加标题和标签
plt.title("Bar Chart Example")
plt.xlabel("X Axis Label")
plt.ylabel("Y Axis Label")
# 显示图像
plt.show()
```
曲面图:
```python
import matplotlib.pyplot as plt
import numpy as np
from mpl_toolkits import mplot3d
# 函数
def f(x, y):
return np.sin(np.sqrt(x ** 2 + y ** 2))
# 数据
x = np.linspace(-6, 6, 30)
y = np.linspace(-6, 6, 30)
X, Y = np.meshgrid(x, y)
Z = f(X, Y)
# 绘制曲面图
fig = plt.figure()
ax = plt.axes(projection='3d')
ax.plot_surface(X, Y, Z, cmap='viridis')
# 添加标题和标签
ax.set_title('Surface Plot Example')
ax.set_xlabel('X Axis Label')
ax.set_ylabel('Y Axis Label')
ax.set_zlabel('Z Axis Label')
# 显示图像
plt.show()
```
阅读全文
相关推荐
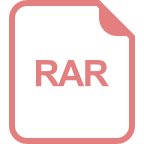
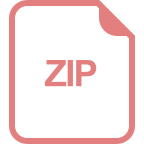
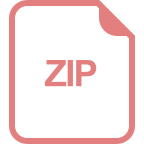
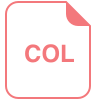





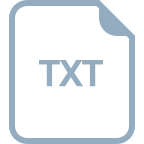
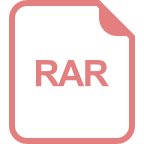
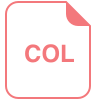



