python:scalar_bar = vtkScalarBarActor(),如何设置scalar_bar的title字体大小和位置
时间: 2024-02-03 18:11:52 浏览: 221
要设置`scalar_bar`的标题字体大小和位置,可以使用以下代码:
```python
# 设置标题字体大小
scalar_bar.SetTitleTextProperty(text_property)
text_property = scalar_bar.GetTitleTextProperty()
text_property.SetFontSize(20)
# 设置标题位置
scalar_bar.SetTitlePosition(title_position)
# title_position是一个四元组(x, y),表示标题位置的坐标,
# 其中x和y都是0到1之间的数值,表示该位置相对于scalar_bar的宽度和高度的比例。
```
其中,`text_property`是`vtkTextProperty`对象,可以用来设置字体的属性,如字体大小、字体颜色等。`title_position`是一个四元组`(x, y)`,表示标题位置的坐标,其中`x`和`y`都是0到1之间的数值,表示该位置相对于`scalar_bar`的宽度和高度的比例。
相关问题
python:scalar_bar = vtkScalarBarActor(),如何设置scalar_bar的title字体大小和位置,比如我想将title的位置设置成和标量条对齐,而不是和数据对齐?
你可以使用vtkTextProperty类设置scalar_bar的title字体大小和位置,代码示例如下:
```python
# 设置scalar_bar的title字体大小
text_property = vtk.vtkTextProperty()
text_property.SetFontSize(16)
scalar_bar.SetTitleTextProperty(text_property)
# 设置scalar_bar的title位置
title_position = scalar_bar.GetPosition()
title_position[0] = title_position[0] + scalar_bar.GetWidth() / 2.0
title_position[1] = title_position[1] + scalar_bar.GetHeight() + 10
scalar_bar.SetTitlePosition(title_position)
```
其中,text_property.SetFontSize(16)设置title字体大小为16,而scalar_bar.SetTitlePosition(title_position)设置title的位置为标量条上方一定距离,title_position变量存储了title的坐标位置。
阅读全文
相关推荐
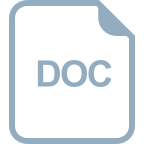
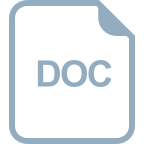
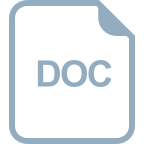
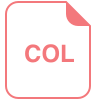
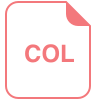
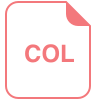
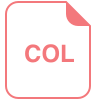
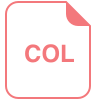
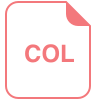
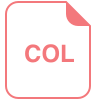
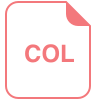
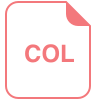
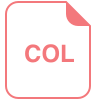
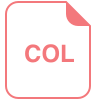
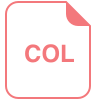
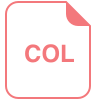
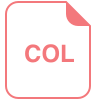