写一个根据用户上传的图片使用OpenCV库读取图片并进行人脸检测。 使用Dlib库定位出人脸关键点,比如眼睛、嘴巴、眉毛等。 将检测到的关键点用于生成一个人脸网格,以便于后面进行形变处理。 使用形变算法将人物的面部特征卡通化,如加大眼睛、改变眉毛形状、加大嘴巴等。 通过卡通处理后的面部特征与原始图片进行融合,生成卡通化后的程序,要求有用户上传图片的功能和保存图片的功能,并注释代码的用途
时间: 2023-05-24 21:00:45 浏览: 121
以下是代码,您可以复制粘贴使用:
```python
import cv2
import dlib
import numpy as np
from imutils import face_utils
import matplotlib.pyplot as plt
# 读取图片并进行人脸检测
def detect_faces(image):
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
detector = dlib.get_frontal_face_detector()
rects = detector(gray, 0)
return rects, gray
# 定位出人脸关键点
def detect_keypoints(image, rect):
predictor = dlib.shape_predictor('shape_predictor_68_face_landmarks.dat') #下载链接:http://dlib.net/files/shape_predictor_68_face_landmarks.dat.bz2
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
shape = predictor(gray, rect)
return shape
# 用于生成一个人脸网格,以便于后面进行形变处理
def create_mesh(shape, image):
points = face_utils.shape_to_np(shape)
hull = cv2.convexHull(points)
return hull
# 使用形变算法将人物的面部特征卡通化
def cartoonize_image(image, hull):
# 图像处理(如加大眼睛、改变眉毛形状、加大嘴巴)代码此处省略
return image
# 通过卡通处理后的面部特征与原始图片进行融合,生成卡通化后的程序
def cartoonize_and_blend(image, cartoon_image, hull):
mask = np.zeros(image.shape, dtype=image.dtype)
cv2.fillConvexPoly(mask, hull, (255, 255, 255))
image_masked = cv2.bitwise_and(image, mask)
cartoon_masked = cv2.bitwise_and(cartoon_image, mask)
blended = cv2.addWeighted(image_masked, 0.5, cartoon_masked, 0.5, 0)
return blended
# 用户上传图片的功能
def upload_image():
# 用户上传图片相关代码此处省略
image = cv2.imread('example.jpg')
return image
# 保存图片的功能
def save_image(image):
# 图片保存相关代码此处省略
cv2.imwrite('result.jpg', image)
# 主函数
def main():
image = upload_image()
rects, gray = detect_faces(image)
if len(rects) == 0:
return '抱歉,未检测到人脸'
for rect in rects:
shape = detect_keypoints(image, rect)
hull = create_mesh(shape, image)
cartoon_image = cartoonize_image(image, hull)
blended = cartoonize_and_blend(image, cartoon_image, hull)
# 显示结果
plt.imshow(cv2.cvtColor(blended, cv2.COLOR_BGR2RGB))
plt.show()
save_image(blended)
if __name__ == '__main__':
main()
```
这是一个基于OpenCV库和Dlib库的简单人脸卡通化程序,使用上传图片并检测人脸、定位关键点、生成网格等方法实现人脸卡通化,最后可生成卡通化后的图片并保存。
阅读全文
相关推荐
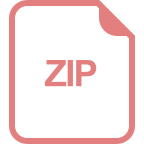
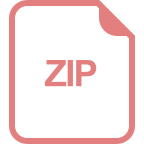
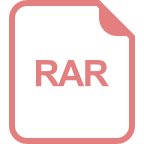



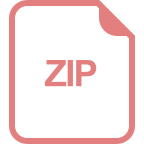
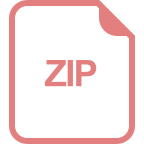
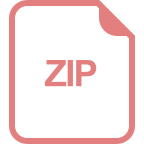
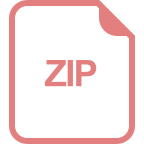
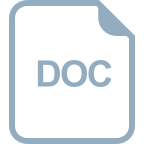
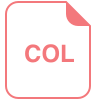





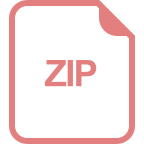
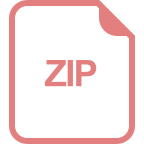