c++显示无向图和有向图的邻接矩阵
时间: 2023-06-19 20:07:18 浏览: 112
以下是 C++ 代码示例,用于显示无向图和有向图的邻接矩阵:
```c++
#include <iostream>
using namespace std;
const int MAX = 100;
// 无向图邻接矩阵
void displayUndirectedGraph(int graph[MAX][MAX], int vertices) {
cout << "无向图邻接矩阵:" << endl;
for (int i = 0; i < vertices; i++) {
for (int j = 0; j < vertices; j++) {
cout << graph[i][j] << " ";
}
cout << endl;
}
}
// 有向图邻接矩阵
void displayDirectedGraph(int graph[MAX][MAX], int vertices) {
cout << "有向图邻接矩阵:" << endl;
for (int i = 0; i < vertices; i++) {
for (int j = 0; j < vertices; j++) {
cout << graph[i][j] << " ";
}
cout << endl;
}
}
int main() {
int vertices, edges, graph[MAX][MAX];
cout << "请输入图的顶点数和边数:" << endl;
cin >> vertices >> edges;
// 初始化邻接矩阵
for (int i = 0; i < vertices; i++) {
for (int j = 0; j < vertices; j++) {
graph[i][j] = 0;
}
}
// 读取边的信息
int src, dest;
for (int i = 0; i < edges; i++) {
cout << "请输入第 " << i + 1 << " 条边的起点和终点:" << endl;
cin >> src >> dest;
graph[src][dest] = 1;
graph[dest][src] = 1; // 无向图需要反向增加一条边
}
// 显示邻接矩阵
displayUndirectedGraph(graph, vertices);
displayDirectedGraph(graph, vertices);
return 0;
}
```
在上面的代码示例中,我们使用了两个函数 `displayUndirectedGraph` 和 `displayDirectedGraph` 来分别显示无向图和有向图的邻接矩阵。通过输入图的顶点数和边数,以及每条边的起点和终点,我们可以构建邻接矩阵,并将其显示出来。其中,对于无向图,我们需要反向增加一条边,以便将边的信息存储在邻接矩阵中。
阅读全文
相关推荐
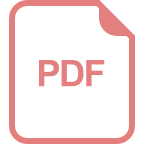
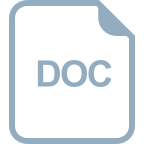
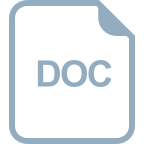
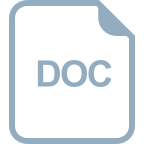
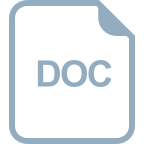











