帮我用python做一个俄罗斯方块小游戏
时间: 2023-10-05 17:09:07 浏览: 102
当然可以,不过这个任务比较复杂,需要一定的编程基础和时间,需要你自己动手实现。以下是一个简单的俄罗斯方块小游戏的代码,你可以参考一下:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口大小
screen_width = 360
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("俄罗斯方块")
# 定义方块大小和颜色
block_size = 30
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
green = (0, 255, 0)
blue = (0, 0, 255)
yellow = (255, 255, 0)
purple = (128, 0, 128)
colors = [red, green, blue, yellow, purple]
# 定义方块类型和形状
shapes = [
[[1, 1, 1],
[0, 1, 0]],
[[0, 2, 2],
[2, 2, 0]],
[[3, 3, 0],
[0, 3, 3]],
[[4, 0, 0],
[4, 4, 4]],
[[0, 0, 5],
[5, 5, 5]],
[[6, 6],
[6, 6]],
[[7, 7, 7, 7]]
]
# 定义游戏区域和边界
play_width = 10 * block_size
play_height = 20 * block_size
play_x = (screen_width - play_width) // 2
play_y = screen_height - play_height - 20
top_left_x = play_x
top_left_y = play_y - 5 * block_size
# 定义方块类
class Block:
def __init__(self, shape):
self.shape = shape
self.color = random.choice(colors)
self.x = top_left_x + play_width // 2 - len(shape[0]) // 2
self.y = top_left_y - len(shape)
self.rotation = 0
def draw(self):
for i in range(len(self.shape)):
for j in range(len(self.shape[0])):
if self.shape[i][j] != 0:
pygame.draw.rect(screen, self.color, (self.x + j * block_size, self.y + i * block_size, block_size, block_size), 0)
def move(self, dx, dy):
self.x += dx * block_size
self.y += dy * block_size
def rotate(self):
self.rotation = (self.rotation + 1) % len(self.shape)
self.shape = rotate_matrix(self.shape)
# 旋转矩阵
def rotate_matrix(matrix):
return [[matrix[j][i] for j in range(len(matrix))] for i in range(len(matrix[0]) - 1, -1, -1)]
# 检查方块是否合法
def is_valid_pos(block, board):
for i in range(len(block.shape)):
for j in range(len(block.shape[0])):
if block.shape[i][j] != 0:
x = block.x + j
y = block.y + i
if x < 0 or x >= play_width // block_size or y < 0 or y >= play_height // block_size or board[y][x] != 0:
return False
return True
# 将方块加入到游戏区域
def add_block_to_board(block, board):
for i in range(len(block.shape)):
for j in range(len(block.shape[0])):
if block.shape[i][j] != 0:
x = block.x + j
y = block.y + i
board[y][x] = block.color
# 消除满行
def clear_lines(board):
lines_cleared = 0
for i in range(len(board)):
if all(block != 0 for block in board[i]):
board.pop(i)
board.insert(0, [0] * (play_width // block_size))
lines_cleared += 1
return lines_cleared
# 绘制游戏区域和方块
def draw_play_area(board, score):
font = pygame.font.SysFont(None, 25)
label = font.render("Score: " + str(score), True, white)
screen.blit(label, (top_left_x, top_left_y - 40))
for i in range(len(board)):
for j in range(len(board[0])):
pygame.draw.rect(screen, board[i][j], (top_left_x + j * block_size, top_left_y + i * block_size, block_size, block_size), 0)
pygame.draw.rect(screen, white, (top_left_x, top_left_y, play_width, play_height), 5)
# 主函数
def main():
board = [[0] * (play_width // block_size) for _ in range(play_height // block_size)]
score = 0
clock = pygame.time.Clock()
block = Block(random.choice(shapes))
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
block.move(-1, 0)
if not is_valid_pos(block, board):
block.move(1, 0)
if event.key == pygame.K_RIGHT:
block.move(1, 0)
if not is_valid_pos(block, board):
block.move(-1, 0)
if event.key == pygame.K_DOWN:
block.move(0, 1)
if not is_valid_pos(block, board):
block.move(0, -1)
if event.key == pygame.K_UP:
block.rotate()
if not is_valid_pos(block, board):
block.rotate()
block.move(0, 1)
if not is_valid_pos(block, board):
block.move(0, -1)
add_block_to_board(block, board)
lines_cleared = clear_lines(board)
score += lines_cleared * 10
block = Block(random.choice(shapes))
if not is_valid_pos(block, board):
pygame.quit()
quit()
screen.fill(black)
draw_play_area(board, score)
block.draw()
pygame.display.update()
clock.tick(10)
# 运行游戏
if __name__ == '__main__':
main()
```
代码中使用了pygame库来实现游戏界面和操作,具体的实现细节可以自行查阅pygame文档。
阅读全文
相关推荐
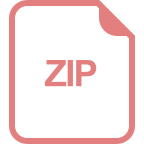











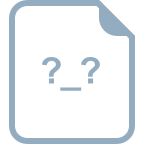