stm32小车pid算法速度闭环控制
时间: 2024-12-26 07:25:32 浏览: 9
### STM32 小车 PID 算法 速度闭环 控制 实现 教程
#### 连接硬件组件
为了实现基于STM32的小车速度闭环控制,首先需要正确连接各个硬件组件。五路循迹传感器应按照如下方式连接至STM32开发板:
| 传感器引脚 | 开发板引脚 |
| --- | --- |
| VCC | 5V |
| GND | GND |
| OUT1 | GPIO PA4 |
| OUT2 | GPIO PA5 |
| OUT3 | GPIO PA6 |
| OUT4 | GPIO PA7 |
| OUT5 | GPIO PB0 |
L293D电机驱动模块用于控制电机转动方向和速度。
#### 初始化配置
初始化阶段主要涉及设置GPIO端口模式以及PWM通道参数。对于PID控制器而言,还需要定义一些必要的变量来保存误差、上一次误差、累积误差等数据结构[^1]。
```c
// 定义全局变量存储当前速度测量值与期望速度设定值
float current_speed = 0;
const float target_speed = 100; // 单位:RPM 或其他适合单位
// 创建PID实例并初始化参数Kp, Ki, Kd
struct pid_controller {
float kp;
float ki;
float kd;
};
void init_pid(struct pid_controller *pid) {
pid->kp = 2.0f;
pid->ki = 0.1f;
pid->kd = 1.0f;
}
```
#### 编写PID计算逻辑
编写一个函数`calculate_pid()`来进行实时调整。该函数接收实际速度作为输入,并返回经过PID调节后的输出值给PWM占空比。
```c
#include "math.h"
float calculate_pid(struct pid_controller *pid, float setpoint, float process_variable){
static float prev_error = 0;
static float integral = 0;
float error = setpoint - process_variable;
// 防止积分饱和
if (integral >= MAX_INTEGRAL || integral <= MIN_INTEGRAL)
integral += error;
float derivative = error - prev_error;
float output = pid->kp*error + pid->ki*integral + pid->kd*derivative;
prev_error = error;
return output;
}
```
#### 设置定时器中断服务程序
为了让PID算法能够稳定工作,在TIMx_IRQHandler()中加入调用`calculate_pid()`的语句,确保每隔固定时间间隔更新一次PWM信号强度。
```c
extern volatile uint8_t TimerCount;
if(__HAL_TIM_GET_FLAG(&htimX, TIM_IT_UPDATE) != RESET){
__HAL_TIM_CLEAR_IT(&htimX, TIM_IT_UPDATE);
if(TimerCount % 10 == 0){ // 每20ms触发一次
Motor_Set(calculate_pid(&pidMotor1Speed, target_speed, get_current_speed()), 0);
TimerCount = 0;
}
}
```
以上代码片段展示了如何利用STM32平台上的外设资源完成基本的速度反馈回路设计。需要注意的是,具体数值可能因应用场景不同而有所差异,建议根据实际情况适当调整PID系数以获得最佳性能表现[^4]。
阅读全文
相关推荐
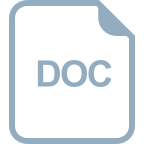
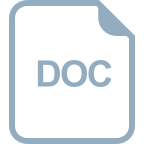
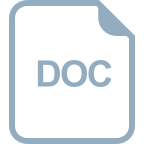


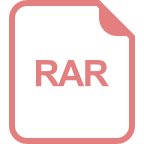
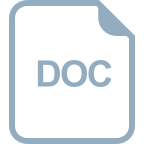











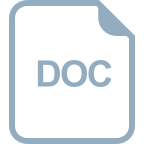
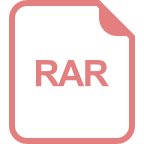