bubble sort
时间: 2023-08-19 08:15:03 浏览: 251
冒泡排序(Bubble Sort)是一种简单的排序算法。它通过重复遍历要排序的数列,每次比较两个相邻的元素,如果它们的顺序错误就交换它们的位置,直到没有需要交换的元素,即数列已经排序完成。冒泡排序的算法描述可以参考以下代码:
```
public static void bubbleSort(int[] arr) {
for(int i = 0; i < arr.length-1; i++) {
for(int j = 0; j < arr.length-i-1; j++) {
if(arr[j] > arr[j+1]) {
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
```
冒泡排序的时间复杂度为最优情况下O(n),表示遍历一次发现没有任何可以交换的元素,排序结束;最坏情况下为O(n^2)。冒泡排序是一种稳定的排序算法,即相等元素的相对位置在排序前后不会发生改变。
相关问题
Bubble Sort
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list to be sorted, compares each adjacent pair of elements and swaps them if they are in the wrong order. This process is repeated until no more swaps are required, indicating that the list is sorted.
The algorithm gets its name from the way smaller elements "bubble" to the top of the list as the algorithm progresses.
Bubble Sort has a time complexity of O(n^2) in the worst case, making it inefficient for large lists. However, it is easy to understand and implement, making it a popular choice for small lists or as an educational tool for teaching sorting algorithms.
python bubble sort
Python 冒泡排序
冒泡排序是一种简单的排序算法,它重复地遍历要排序的列表,比较每对相邻的项,并在必要时交换它们的位置。通过多次遍历列表,将最大的项逐渐“浮”到列表的顶部,因此称为冒泡排序。
以下是 Python 中实现冒泡排序的示例代码:
```python
def bubble_sort(arr):
n = len(arr)
# 遍历所有数组元素
for i in range(n):
# 最后 i 个元素已经排好序
for j in range(0, n-i-1):
# 如果当前元素大于下一个元素,则交换它们的位置
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
# 测试代码
arr = [64, 34, 25, 12, 22, 11, 90]
bubble_sort(arr)
print("排序后的数组:")
for i in range(len(arr)):
print("%d" %arr[i])
```
输出结果:
```
排序后的数组:
11
12
22
25
34
64
90
```
在上面的代码中,我们使用两个嵌套的循环来遍历列表中的所有元素。外部循环用于遍历列表中的所有元素,而内部循环用于比较相邻的元素并交换它们的位置。在每次内部循环结束时,最大的元素都会“浮”到列表的顶部。重复这个过程,直到整个列表都被排序。
阅读全文
相关推荐
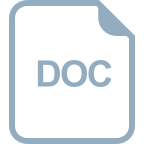
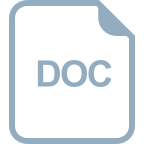
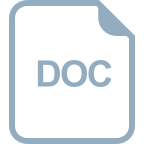



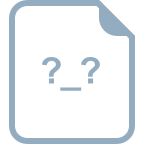
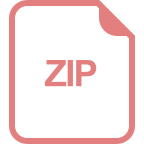
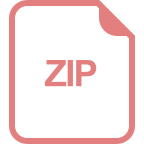
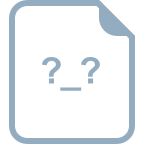
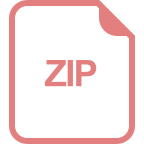


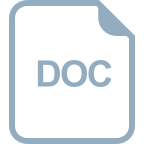
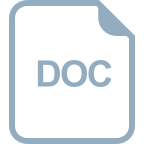