ROS如何在回调函数中打开另一个节点
时间: 2023-06-13 20:04:45 浏览: 145
在ROS中,每个节点都是独立的进程,它们通过ROS Master进行通信。如果一个节点需要与另一个节点交互,可以使用ROS服务、话题或者参数服务器等ROS机制。
如果你需要在回调函数中打开另一个节点,可以使用ROS命令行工具 roslaunch 和 roslaunch API。具体步骤如下:
1. 在ROS包中创建一个启动文件,例如launch_file.launch。在启动文件中定义需要启动的节点,以及节点的参数等信息。
2. 在回调函数中,使用roslaunch API启动另一个节点。例如,使用以下代码启动名为"node2"的节点:
```
roslaunch_api::RoslaunchAPI api;
api.startNode("node2", "/path/to/launch_file.launch");
```
其中,"node2"是要启动的节点名称,"/path/to/launch_file.launch"是启动文件的路径。
需要注意的是,启动节点的代码应该在主线程中执行,以免阻塞回调函数。
除了使用roslaunch API,你还可以使用系统调用函数system()来启动另一个节点,例如:
```
system("roslaunch my_package my_launch_file.launch");
```
这种方法比较简单,但是不够灵活,而且可能会受到安全限制。因此,建议使用roslaunch API来启动节点。
相关问题
ROS如何在节点中打开另一个节点
在ROS中,节点之间通过ROS Master进行通信,一个节点可以通过发送消息的方式与另一个节点进行交互。如果你想要在一个节点中启动另一个节点,可以使用ROS提供的roslaunch命令。
在roslaunch文件中,你可以声明要启动的节点,并指定它们的参数和命名空间。例如,以下是一个roslaunch文件的示例,用于启动两个节点:
```
<launch>
<node name="node1" pkg="package1" type="node1_type" />
<node name="node2" pkg="package2" type="node2_type" />
</launch>
```
当你运行此roslaunch文件时,它将启动“node1”和“node2”节点,并在ROS Master上注册它们。在节点1中,你可以使用ROS提供的rospy库或roscpp库发送消息到节点2,或者订阅来自节点2的消息。例如,以下是一个使用rospy库向节点2发送消息的示例:
```
import rospy
from std_msgs.msg import String
rospy.init_node('node1')
pub = rospy.Publisher('topic_name', String, queue_size=10)
pub.publish('Hello world!')
```
在节点2中,你可以使用相同的库订阅来自节点1的消息,并执行相应的操作。例如,以下是一个使用rospy库订阅来自节点1的消息的示例:
```
import rospy
from std_msgs.msg import String
def callback(data):
rospy.loginfo(rospy.get_caller_id() + 'I heard %s', data.data)
rospy.init_node('node2')
sub = rospy.Subscriber('topic_name', String, callback)
rospy.spin()
```
在此示例中,当节点1向“topic_name”主题发送消息时,节点2将接收到该消息,然后调用回调函数callback,该函数将打印消息内容。
帮我写一个ros2节点,要求使用c++编程,回调函数要求将来自/apriltag_detections 话题的标定数据,即position 和 orientation,保存写入本地文件
好的,下面是一个示例代码,用于在ROS2下订阅apriltag检测结果,并将标定数据保存到本地文件中。
```cpp
#include <iostream>
#include <fstream>
#include "rclcpp/rclcpp.hpp"
#include "apriltag_msgs/msg/apriltag_detection_array.hpp"
#include "geometry_msgs/msg/pose_stamped.hpp"
class AprilTagSubscriber : public rclcpp::Node
{
public:
AprilTagSubscriber() : Node("apriltag_subscriber")
{
// 创建订阅者,订阅apriltag检测结果
subscriber_ = this->create_subscription<apriltag_msgs::msg::AprilTagDetectionArray>(
"/apriltag_detections", 10, std::bind(&AprilTagSubscriber::callback, this, std::placeholders::_1));
// 打开文件,用于保存标定数据
out_file_.open("apriltag_pose.txt");
}
private:
void callback(const apriltag_msgs::msg::AprilTagDetectionArray::SharedPtr msg)
{
// 遍历检测结果,将标定数据保存到文件中
for (const auto& detection : msg->detections)
{
const auto& pose = detection.pose.pose;
out_file_ << "ID: " << detection.id[0] << std::endl;
out_file_ << "Position: " << pose.position.x << " " << pose.position.y << " " << pose.position.z << std::endl;
out_file_ << "Orientation: " << pose.orientation.x << " " << pose.orientation.y << " "
<< pose.orientation.z << " " << pose.orientation.w << std::endl;
}
}
rclcpp::Subscription<apriltag_msgs::msg::AprilTagDetectionArray>::SharedPtr subscriber_;
std::ofstream out_file_;
};
int main(int argc, char* argv[])
{
rclcpp::init(argc, argv);
rclcpp::spin(std::make_shared<AprilTagSubscriber>());
rclcpp::shutdown();
return 0;
}
```
在上面的代码中,我们定义了一个名为`AprilTagSubscriber`的类,该类继承自`rclcpp::Node`。在类的构造函数中,我们创建了一个订阅者,用于订阅`/apriltag_detections`话题上的apriltag检测结果。同时,我们也打开了一个文件,用于保存标定数据。
在订阅到消息后,我们遍历检测结果,并将标定数据保存到文件中。需要注意的是,我们使用了`std::ofstream`来打开文件,并使用`<<`运算符将数据写入文件中。
需要注意的是,在实际使用中,我们需要对保存的文件格式进行规范,以便后续的数据处理和分析。同时,我们也需要注意文件的写入权限和路径问题。
阅读全文
相关推荐
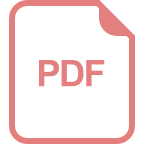
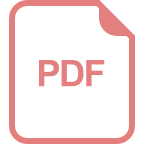
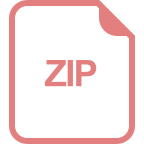
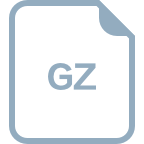
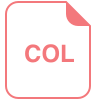










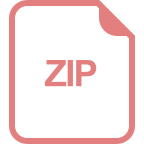