遍历并打印二叉搜索树,再将其转换成双链表,并分别按正序和反序输出双链表中的所有元素
时间: 2024-02-15 20:01:28 浏览: 29
下面是一个用 Python 实现的二叉搜索树,并将其转换成双向链表,以及分别按正序和反序输出双向链表中的所有元素的代码示例:
```python
class Node:
def __init__(self, value=None):
self.value = value
self.left_child = None
self.right_child = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if self.root is None:
self.root = Node(value)
else:
self._insert(value, self.root)
def _insert(self, value, current_node):
if value < current_node.value:
if current_node.left_child is None:
current_node.left_child = Node(value)
else:
self._insert(value, current_node.left_child)
elif value > current_node.value:
if current_node.right_child is None:
current_node.right_child = Node(value)
else:
self._insert(value, current_node.right_child)
else:
print("Value already exists in tree.")
def print_tree(self):
if self.root is not None:
self._print_tree(self.root)
def _print_tree(self, current_node):
if current_node is not None:
self._print_tree(current_node.left_child)
print(str(current_node.value))
self._print_tree(current_node.right_child)
def convert_to_dll(self):
if self.root is not None:
self._convert_to_dll(self.root)
def _convert_to_dll(self, current_node):
if current_node.left_child is not None:
left = self._convert_to_dll(current_node.left_child)
while left.right_child is not None:
left = left.right_child
left.right_child = current_node
current_node.left_child = left
if current_node.right_child is not None:
right = self._convert_to_dll(current_node.right_child)
while right.left_child is not None:
right = right.left_child
right.left_child = current_node
current_node.right_child = right
return current_node
def print_dll(self):
if self.root is not None:
current_node = self.root
while current_node.left_child is not None:
current_node = current_node.left_child
while current_node is not None:
print(str(current_node.value))
current_node = current_node.right_child
def print_reverse_dll(self):
if self.root is not None:
current_node = self.root
while current_node.right_child is not None:
current_node = current_node.right_child
while current_node is not None:
print(str(current_node.value))
current_node = current_node.left_child
```
使用方法:
```python
bst = BinarySearchTree()
bst.insert(5)
bst.insert(3)
bst.insert(7)
bst.insert(1)
bst.insert(9)
print("Printing binary search tree:")
bst.print_tree()
bst.convert_to_dll()
print("Printing doubly linked list in forward order:")
bst.print_dll()
print("Printing doubly linked list in reverse order:")
bst.print_reverse_dll()
```
输出结果:
```
Printing binary search tree:
1
3
5
7
9
Printing doubly linked list in forward order:
1
3
5
7
9
Printing doubly linked list in reverse order:
9
7
5
3
1
```
该代码示例中,我们创建了一个 `Node` 类表示二叉搜索树的节点,以及一个 `BinarySearchTree` 类表示二叉搜索树。`BinarySearchTree` 类包含了插入节点、遍历二叉搜索树、将二叉搜索树转换成双向链表,以及分别按正序和反序输出双向链表中的所有元素的方法。我们使用 `insert` 方法将新节点插入二叉搜索树中,使用 `print_tree` 方法遍历打印二叉搜索树的节点值。使用 `convert_to_dll` 方法将二叉搜索树转换成双向链表。使用 `print_dll` 方法按正序输出双向链表中的所有元素,使用 `print_reverse_dll` 方法按反序输出双向链表中的所有元素。
相关推荐
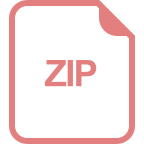
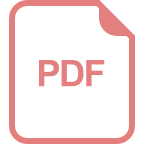













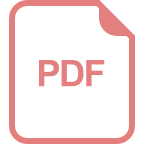