c++汉诺塔不用递归怎么实现
时间: 2024-01-27 07:03:08 浏览: 61
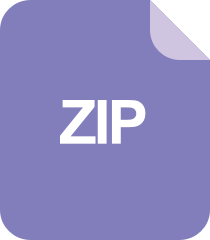
汉诺塔的非递归实现,c++
汉诺塔问题是一个经典的递归问题,但是也可以使用循环来解决。下面是一个使用栈来模拟递归的实现:
```c++
#include <iostream>
#include <stack>
using namespace std;
void hanoi(int n, char A, char B, char C) {
stack<int> s1, s2, s3;
for (int i = n; i >= 1; --i) {
s1.push(i);
}
while (true) {
while (!s1.empty() && (s2.empty() || s1.top() < s2.top())) {
s2.push(s1.top());
s1.pop();
cout << "Move " << s2.top() << " from " << A << " to " << B << endl;
}
while (!s2.empty() && (s3.empty() || s2.top() < s3.top())) {
s3.push(s2.top());
s2.pop();
cout << "Move " << s3.top() << " from " << B << " to " << C << endl;
}
if (s3.size() == n) {
break;
}
while (!s3.empty() && (s2.empty() || s3.top() < s2.top())) {
s2.push(s3.top());
s3.pop();
cout << "Move " << s2.top() << " from " << C << " to " << B << endl;
}
while (!s2.empty() && (s1.empty() || s2.top() < s1.top())) {
s1.push(s2.top());
s2.pop();
cout << "Move " << s1.top() << " from " << B << " to " << A << endl;
}
}
}
int main() {
int n = 3;
hanoi(n, 'A', 'B', 'C');
return 0;
}
```
这个实现将每个柱子看作一个栈,并使用一个 while 循环来模拟递归的过程。每个 while 循环都模拟了递归函数中的一个分支。在每个分支中,栈顶元素会被移动到下一个柱子上,并输出相应的信息。最后,当目标柱子上的栈的大小等于 n 时,就说明所有的盘子都已经移动到了目标柱子上,程序结束。
阅读全文
相关推荐

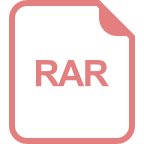
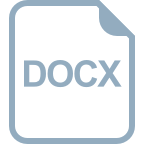
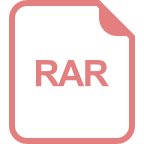
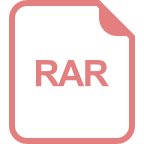
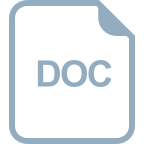
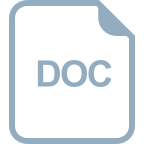
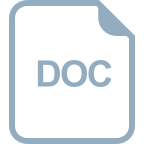





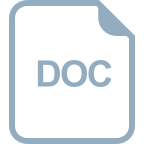