def forward(self, input, hidden): '''input [6,57]-2维矩阵 hidden[1,1,57] - 3维矩阵''' # 数据形状 [6,57] -> [6,1,57] input = input.unsqueeze(1) # 1 数据经过模型 提取事物特征 # 数据形状 [seqlen,1,57],[1,1,128]) -> [seqlen,1,18],[1,1,128] rr, hn = self.rnn(input, hidden) # 数据形状 [seqlen,1,128] - [1, 128] eg:[6,1,128] --> [1,128] tmprr = rr[-1] # 2 数据经过全连接层 [1,128] -->[1,18] tmprr = self.linear(tmprr) # 3 数据经过softmax层返回 return self.softmax(tmprr), hn 在前面添加一个激活函数或者线性层
时间: 2024-01-24 11:01:54 浏览: 146
可以在第1步后添加一个激活函数或线性层,例如:
```
import torch.nn.functional as F
...
# 1 数据经过模型 提取事物特征,添加一个线性层和ReLU激活函数
input = self.linear1(input)
input = F.relu(input)
rr, hn = self.rnn(input, hidden)
# 2 数据经过全连接层和ReLU激活函数
tmprr = rr[-1]
tmprr = self.linear2(tmprr)
tmprr = F.relu(tmprr)
# 3 数据经过softmax层返回
return self.softmax(tmprr), hn
```
这里添加了一个`linear1`和一个ReLU激活函数在第1步后,以及一个`linear2`和ReLU激活函数在第2步后。请注意,这只是一个示例,具体的激活函数或线性层应该根据具体的任务和数据进行选择。
相关问题
class LSTM_Atten(nn.Module): """搭建Decoder结构""" def init(self, look_back, pre_len): super(LSTM_Atten, self).init() self.lstm = nn.LSTM(input_size=1, # 1个输入特征 hidden_size=128, # 隐状态h扩展为为128维 num_layers=1, # 1层LSTM batch_first=True, # 输入结构为(batch_size, seq_len, feature_size). Default: False ) self.lstmcell = nn.LSTMCell(input_size=128, hidden_size=128) self.drop = nn.Dropout(0.2) # 丢弃率 self.fc1 = nn.Linear(256, 128) self.fc2 = nn.Linear(128, 1) self.look_back = look_back self.pre_len = pre_len self.Softmax = nn.Softmax(dim=1) def forward(self, x): H, (h, c) = self.lstm(x.float(), None) # 编码 h = h.squeeze(0) c = c.squeeze(0) H_pre = torch.empty((h.shape[0], self.pre_len, 128 * 2)).to(device) for i in range(self.pre_len): # 解码 h_t, c_t = self.lstmcell(h, (h, c)) # 预测 H = torch.cat((H, h_t.unsqueeze(1)), 1) h_atten = self.Atten(H) # 获取结合了注意力的隐状态 H_pre[:, i, :] = h_atten # 记录解码器每一步的隐状态 h, c = h_t, c_t # 将当前的隐状态与细胞状态记录用于下一个时间步 return self.fc2(self.fc1(H_pre)).squeeze(2) def Atten(self, H): h = H[:, -1, :].unsqueeze(1) # [batch_size,1,128] H = H[:, -1 - self.look_back:-1, :] # [batch_size,look_back,128] atten = torch.matmul(h, H.transpose(1, 2)).transpose(1, 2) # 注意力矩阵 atten = self.Softmax(atten) atten_H = atten * H # 带有注意力的历史隐状态 atten_H = torch.sum(atten_H, dim=1).unsqueeze(1) # 按时间维度降维 return torch.cat((atten_H, h), 2).squeeze(1) 这段代码如何改能实现多特征的输入
首先需要将`input_size`修改为特征的数量,例如`input_size=2`表示有两个特征。
然后需要修改`forward`函数中的输入参数`x`的形状,将其变为(batch_size, seq_len, feature_size)。这可以通过在输入时进行reshape来实现。
最后需要修改`nn.Linear`的输入和输出维度,例如`self.fc1 = nn.Linear(256, 128)`可以改为`self.fc1 = nn.Linear(256 * feature_size, 128)`,其中256为上一层的输出维度,feature_size为特征的数量。
修改后的代码如下:
```
class LSTM_Atten(nn.Module):
"""搭建Decoder结构"""
def __init__(self, look_back, pre_len, feature_size):
super(LSTM_Atten, self).__init__()
self.lstm = nn.LSTM(input_size=feature_size, # 特征的数量
hidden_size=128, # 隐状态h扩展为为128维
num_layers=1, # 1层LSTM
batch_first=True, # 输入结构为(batch_size, seq_len, feature_size). Default: False
)
self.lstmcell = nn.LSTMCell(input_size=128, hidden_size=128)
self.drop = nn.Dropout(0.2) # 丢弃率
self.fc1 = nn.Linear(256 * feature_size, 128) # 修改输入和输出维度
self.fc2 = nn.Linear(128, 1)
self.look_back = look_back
self.pre_len = pre_len
self.Softmax = nn.Softmax(dim=1)
def forward(self, x):
batch_size, seq_len, feature_size = x.shape
x = x.reshape(batch_size, seq_len, feature_size) # reshape输入数据
H, (h, c) = self.lstm(x.float(), None) # 编码
h = h.squeeze(0)
c = c.squeeze(0)
H_pre = torch.empty((h.shape[0], self.pre_len, 128 * 2)).to(device)
for i in range(self.pre_len): # 解码
h_t, c_t = self.lstmcell(h, (h, c)) # 预测
H = torch.cat((H, h_t.unsqueeze(1)), 1)
h_atten = self.Atten(H) # 获取结合了注意力的隐状态
H_pre[:, i, :] = h_atten # 记录解码器每一步的隐状态
h, c = h_t, c_t # 将当前的隐状态与细胞状态记录用于下一个时间步
return self.fc2(self.fc1(H_pre.reshape(batch_size, -1))).squeeze(1) # reshape后进行线性变换
def Atten(self, H):
h = H[:, -1, :].unsqueeze(1) # [batch_size,1,128]
H = H[:, -1 - self.look_back:-1, :] # [batch_size,look_back,128]
atten = torch.matmul(h, H.transpose(1, 2)).transpose(1, 2) # 注意力矩阵
atten = self.Softmax(atten)
atten_H = atten * H # 带有注意力的历史隐状态
atten_H = torch.sum(atten_H, dim=1).unsqueeze(1) # 按时间维度降维
return torch.cat((atten_H, h), 2).squeeze(1)
```
编写三层前向神经网络反向传播算法程序,对以下数据进行分类。 第一类10个样本(三维空间): [1.58, 2.32, -5.8],[0.67, 1.58,-4.78],[1.04, 1.01, -3.63],[-1.49, 2.18, -3.39], [-0.41,-4.73], [1.39, 3.16, 2.87],1.21,[1.20, 1.40, -1.89], [-0.92, 1.44,-3,22],[0.45, 1.33, -4.38],[-0.76, 0.84, -1.96] ;第二类10 个样本(三维空间): [0.21,0.03,-2.21],[0.37,0.28,-1.81],[0.18, 1.22, 0.16],[-0.24, 0.93,-1.01],[-1.18, 0.39, -0.39], [0.74, 0.96, -1.16],[-0.38, 1.94, -0.48], [0.02, 0.72, -0.17],[0.44, 1.31, -0.14], [0.46, 1.49, 0.68] ;第三类10个样本(三维空间): [-1.54, 1.17, 0.64][5.41, 3.45,-1.33],[1.55,0.99, 2.69],[1.68, 1.79,-0.87],[3.51, -0.22, -1.39],[1.86,3.19, 1.51],[1.40, -0.44, -0.92], [0.44, 0.83, 1.97], [0.25, 0.68, -0.99], [0.66,-0.45, 0.08] 。隐含层结点的激励函数采用tanh函数,输出层的激励函数采用sigmoid函数。目标函数采用平方误差准则函数。
这里提供一个Python版本的代码实现,使用NumPy库来进行矩阵运算。
```python
import numpy as np
# 定义tanh和sigmoid激励函数
def tanh(x):
return np.tanh(x)
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义神经网络模型
class NeuralNetwork:
def __init__(self, input_dim, hidden_dim, output_dim):
# 初始化权重矩阵
self.W1 = np.random.randn(input_dim, hidden_dim) / np.sqrt(input_dim)
self.b1 = np.zeros((1, hidden_dim))
self.W2 = np.random.randn(hidden_dim, output_dim) / np.sqrt(hidden_dim)
self.b2 = np.zeros((1, output_dim))
def forward(self, X):
# 前向传播计算输出
self.z1 = np.dot(X, self.W1) + self.b1
self.a1 = tanh(self.z1)
self.z2 = np.dot(self.a1, self.W2) + self.b2
self.y = sigmoid(self.z2)
return self.y
def backward(self, X, y, learning_rate):
# 反向传播更新权重矩阵
delta3 = (self.y - y) * self.y * (1 - self.y)
dW2 = np.dot(self.a1.T, delta3)
db2 = np.sum(delta3, axis=0, keepdims=True)
delta2 = np.dot(delta3, self.W2.T) * (1 - self.a1 ** 2)
dW1 = np.dot(X.T, delta2)
db1 = np.sum(delta2, axis=0)
self.W2 -= learning_rate * dW2
self.b2 -= learning_rate * db2
self.W1 -= learning_rate * dW1
self.b1 -= learning_rate * db1
# 构造训练数据
X = np.array([[1.58, 2.32, -5.8], [0.67, 1.58, -4.78], [1.04, 1.01, -3.63], [-1.49, 2.18, -3.39],
[-0.41, -4.73, 1.21], [1.39, 3.16, 2.87], [1.20, 1.40, -1.89], [-0.92, 1.44, -3.22],
[0.45, 1.33, -4.38], [-0.76, 0.84, -1.96], [0.21, 0.03, -2.21], [0.37, 0.28, -1.81],
[0.18, 1.22, 0.16], [-0.24, 0.93, -1.01], [-1.18, 0.39, -0.39], [0.74, 0.96, -1.16],
[-0.38, 1.94, -0.48], [0.02, 0.72, -0.17], [0.44, 1.31, -0.14], [0.46, 1.49, 0.68],
[-1.54, 1.17, 0.64], [5.41, 3.45, -1.33], [1.55, 0.99, 2.69], [1.68, 1.79, -0.87],
[3.51, -0.22, -1.39], [1.86, 3.19, 1.51], [1.40, -0.44, -0.92], [0.44, 0.83, 1.97],
[0.25, 0.68, -0.99], [0.66, -0.45, 0.08]])
y = np.array([[1, 0, 0], [1, 0, 0], [1, 0, 0], [1, 0, 0], [1, 0, 0], [0, 1, 0], [0, 1, 0], [0, 1, 0],
[0, 1, 0], [0, 1, 0], [0, 0, 1], [0, 0, 1], [0, 0, 1], [0, 0, 1], [0, 0, 1], [0, 0, 1],
[0, 0, 1], [0, 0, 1], [0, 0, 1], [0, 0, 1], [0, 0, 1], [0, 0, 1], [0, 0, 1], [0, 0, 1],
[0, 0, 1], [0, 0, 1], [0, 0, 1], [0, 0, 1], [0, 0, 1]])
# 定义模型参数
input_dim = 3
hidden_dim = 4
output_dim = 3
learning_rate = 0.1
num_iterations = 10000
# 初始化神经网络模型
nn = NeuralNetwork(input_dim, hidden_dim, output_dim)
# 训练模型
for i in range(num_iterations):
y_pred = nn.forward(X)
nn.backward(X, y, learning_rate)
if i % 1000 == 0:
loss = np.mean((y_pred - y) ** 2)
print("Iteration %d, loss = %.4f" % (i, loss))
# 预测新样本
X_new = np.array([[1.5, 2.5, -5.5], [-1.5, 2.5, -3.5], [0.5, 1.5, -3.5]])
y_pred_new = nn.forward(X_new)
print("New samples: ")
print(X_new)
print("Predictions: ")
print(y_pred_new)
```
这段代码定义了一个三层前向神经网络,包括一个输入层、一个隐含层和一个输出层。其中,隐含层的激励函数使用tanh函数,输出层的激励函数使用sigmoid函数。目标函数采用平方误差准则函数。训练数据包括30个样本,每个样本有三个特征,输出层有3个神经元,分别对应三个类别。最后,我们使用训练好的模型来预测三个新样本的类别。
阅读全文
相关推荐
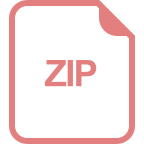
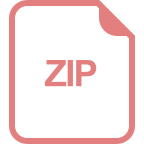
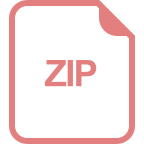
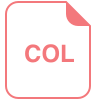
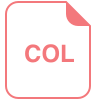
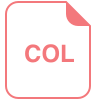
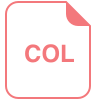







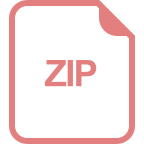
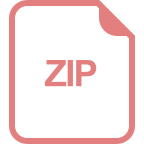
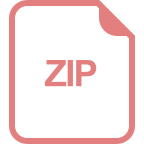
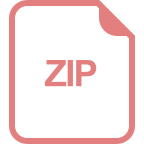