利用python库pyrealsense2实现按下按钮保存当前点云,格式为ply带颜色,不用open3D库
时间: 2024-03-02 15:51:20 浏览: 236
好的,以下是不使用Open3D库实现按下按钮保存当前点云,格式为ply带颜色的代码:
```python
import pyrealsense2 as rs
import numpy as np
# Configure depth and color streams
pipeline = rs.pipeline()
config = rs.config()
config.enable_stream(rs.stream.depth, 640, 480, rs.format.z16, 30)
config.enable_stream(rs.stream.color, 640, 480, rs.format.bgr8, 30)
# Start streaming
pipeline.start(config)
try:
while True:
# Wait for a coherent pair of frames: depth and color
frames = pipeline.wait_for_frames()
depth_frame = frames.get_depth_frame()
color_frame = frames.get_color_frame()
if not depth_frame or not color_frame:
continue
# Convert images to numpy arrays
depth_image = np.asanyarray(depth_frame.get_data())
color_image = np.asanyarray(color_frame.get_data())
# Create point cloud from depth image and color image
pc = rs.pointcloud()
points = pc.calculate(depth_frame)
pc.map_to(color_frame)
colors = np.asanyarray(color_frame.get_data())
verts = np.asanyarray(points.get_vertices())
# Save point cloud to ply file with colors
file_name = 'point_cloud.ply'
with open(file_name, 'w') as f:
f.write('ply\n')
f.write('format ascii 1.0\n')
f.write('element vertex {}\n'.format(verts.shape[0]))
f.write('property float x\n')
f.write('property float y\n')
f.write('property float z\n')
f.write('property uchar red\n')
f.write('property uchar green\n')
f.write('property uchar blue\n')
f.write('end_header\n')
for i in range(verts.shape[0]):
point = verts[i]
color = colors[i]
x, y, z = point
r, g, b = color
f.write('{:.4f} {:.4f} {:.4f} {} {} {}\n'.format(x, y, z, r, g, b))
except KeyboardInterrupt:
pass
finally:
# Stop streaming
pipeline.stop()
```
在上述代码中,我们使用了Python内置的文件操作函数来保存点云到ply文件,并且将颜色信息也保存在了文件中。您可以根据自己的需求修改保存文件的路径和文件名。注意,这里写入文件时使用了“w”模式,这将会覆盖原来的文件,如果需要追加数据到文件末尾,可以使用“a”模式。
阅读全文
相关推荐
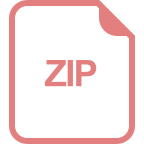
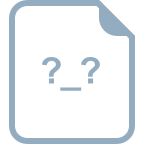
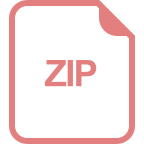















