x_direction = choice([1,-1]) x_distance = choice([0,1,2,3,4]) x_step = x_direction*x_distance
时间: 2023-10-24 12:04:39 浏览: 40
这段代码使用了Python中的random模块的choice函数来随机选择x_direction和x_distance的值。x_direction被选择为1或-1,用于决定x_step的方向。x_distance被选择为0、1、2、3或4,用于决定x_step的距离。最后,x_step被计算为x_direction乘以x_distance,得到了最终的步长。
相关问题
Python绘制随机漫步图
下面是一个简单的 Python 代码,可以使用 matplotlib 库绘制一个随机漫步图:
```python
import matplotlib.pyplot as plt
from random import choice
class RandomWalk:
def __init__(self, num_points=5000):
self.num_points = num_points
self.x_values = [0]
self.y_values = [0]
def fill_walk(self):
while len(self.x_values) < self.num_points:
x_direction = choice([1, -1])
x_distance = choice([0, 1, 2, 3, 4])
x_step = x_direction * x_distance
y_direction = choice([1, -1])
y_distance = choice([0, 1, 2, 3, 4])
y_step = y_direction * y_distance
if x_step == 0 and y_step == 0:
continue
next_x = self.x_values[-1] + x_step
next_y = self.y_values[-1] + y_step
self.x_values.append(next_x)
self.y_values.append(next_y)
rw = RandomWalk()
rw.fill_walk()
plt.figure(dpi=128, figsize=(10, 6))
plt.plot(rw.x_values, rw.y_values, linewidth=1)
plt.show()
```
这个代码使用 RandomWalk 类来生成随机漫步的坐标,并使用 matplotlib 库将其绘制出来。运行代码后,应该会生成一个随机漫步图。
python3 random.randomrange_Python 3实现随机漫步(Random Walk)
随机漫步是一种经常用于模拟随机过程的数学模型,可以用于模拟股票价格、天气变化等随机变化的过程。下面是一个 Python 3 实现随机漫步的例子:
```python
import random
import matplotlib.pyplot as plt
# 设置起始点
x = 0
y = 0
# 设置步数
steps = 1000
# 定义两个数组来存储每一步的 x 和 y 坐标
x_values = [0]
y_values = [0]
# 在指定步数内进行随机漫步
for i in range(steps):
# 随机选择方向和距离
direction = random.choice([-1, 1])
distance = random.randint(0, 5)
# 计算下一步的 x 和 y 坐标
x += direction * distance
y += direction * distance
# 将下一步的坐标存储到数组中
x_values.append(x)
y_values.append(y)
# 绘制随机漫步图像
plt.plot(x_values, y_values, linewidth=2)
plt.title("Random Walk")
plt.xlabel("X", fontsize=14)
plt.ylabel("Y", fontsize=14)
plt.tick_params(axis='both', which='major', labelsize=14)
plt.show()
```
代码中,我们首先设置起始点 `(0, 0)` 和步数 `1000`。然后在 `for` 循环中进行随机漫步,每一步随机选择一个方向和距离。最后,将每一步的 x 和 y 坐标存储到对应的数组中,并使用 `matplotlib` 绘制出随机漫步的图像。运行上述代码可以得到类似下图的随机漫步图像:

相关推荐
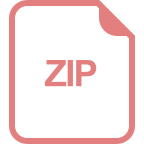
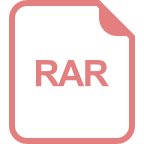












