解释代码 for line in lines: rho, theta = line[0] angle = theta * 180 / np.pi
时间: 2024-02-14 19:27:55 浏览: 31
这段代码是在一个循环中遍历变量 `lines`,`lines` 是通过霍夫变换检测图像中的直线得到的结果。在每次迭代中,`line` 代表着一条检测到的直线。
在代码中,使用 `line[0]` 来获取每条直线的参数 `rho` 和 `theta`。`rho` 是直线在极坐标中的距离原点的距离,而 `theta` 是直线在极坐标中的角度。
接下来,通过将 `theta` 乘以 180 再除以 π,将角度转换为以度为单位的值。这样可以得到每条直线的角度,即 `angle`。
此段代码的目的可能是为了计算每条直线的角度,并进行进一步处理或分析。
相关问题
def process_image(image_path, output_folder): gray = cv2.imread(image_path) edges = cv2.Canny(gray, 50, 150) lines = cv2.HoughLines(edges, 1, np.pi / 180, threshold=90) sum_angle = 0 count = 0 if lines is not None: for line in lines: rho, theta = line[0] angle = theta * 180 / np.pi sum_angle += angle count += 1 avg_angle = sum_angle / count rotated_image = rotate_image(gray, avg_angle) filename = os.path.basename(image_path) output_path = os.path.join(output_folder, filename) cv2.imwrite(output_path, rotated_image) def rotate_image(image, angle): height, width = image.shape[:2] center = (width // 2, height // 2) rotation_matrix = cv2.getRotationMatrix2D(center, angle, 1.0) rotated_image = cv2.warpAffine(image, rotation_matrix, (width, height)) return rotated_image input_folder = 'img_out_binary' output_folder = 'rotated_img' os.makedirs(output_folder, exist_ok=True) for filename in os.listdir(input_folder): if filename.endswith(".jpg") or filename.endswith(".JPG"): image_path = os.path.join(input_folder, filename) process_image(image_path, output_folder) 优化程序,使程序可以对图片文件名包含汉字的图片进行处理和保存
要使程序能够处理和保存包含汉字的图片文件名,您可以对`process_image()`函数进行修改。在处理图片之前,您可以对文件名进行URL编码,然后使用编码后的文件名来保存旋转后的图片。以下是修改后的代码:
```python
def process_image(image_path, output_folder):
# 对文件名进行URL编码
encoded_filename = urllib.parse.quote(os.path.basename(image_path))
gray = cv2.imread(image_path)
edges = cv2.Canny(gray, 50, 150)
lines = cv2.HoughLines(edges, 1, np.pi / 180, threshold=90)
sum_angle = 0
count = 0
if lines is not None:
for line in lines:
rho, theta = line[0]
angle = theta * 180 / np.pi
sum_angle += angle
count += 1
avg_angle = sum_angle / count
rotated_image = rotate_image(gray, avg_angle)
# 使用编码后的文件名来保存旋转后的图片
output_path = os.path.join(output_folder, encoded_filename)
cv2.imwrite(output_path, rotated_image)
```
在修改后的代码中,首先使用`urllib.parse.quote()`函数对文件名进行编码,然后使用编码后的文件名来构建输出路径。这样可以确保保存的文件名不会受到包含汉字的影响。
另外,确保在程序中正确导入`urllib.parse`模块来使用URL编码函数。
更正代码,因为代码出现以下异常:cv2.error: OpenCV(3.4.8) C:\projects\opencv-python\opencv\modules\imgproc\src\color.cpp:182: error: (-215:Assertion failed) !_src.empty() in function 'cv::cvtColor'
好的,以下是更正后的代码:
```python
import cv2
import numpy as np
# 读取原始图像
img = cv2.imread('image.jpg')
if img is None: # 判断图像是否读取成功
print('Failed to read image!')
else:
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 边缘检测
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
# 获取直线
lines = cv2.HoughLines(edges, 1, np.pi/180, 200)
# 计算角度
angles = []
for line in lines:
for rho, theta in line:
a = np.cos(theta)
b = np.sin(theta)
x0 = a*rho
y0 = b*rho
x1 = int(x0 + 1000*(-b))
y1 = int(y0 + 1000*(a))
x2 = int(x0 - 1000*(-b))
y2 = int(y0 - 1000*(a))
angle = np.arctan2(y2-y1, x2-x1)
angles.append(angle)
# 计算平均角度
avg_angle = np.mean(angles)
# 旋转校正
rows, cols = img.shape[:2]
M = cv2.getRotationMatrix2D((cols/2, rows/2), avg_angle*180/np.pi, 1)
corrected_img = cv2.warpAffine(img, M, (cols, rows))
# 显示结果
cv2.imshow('Original Image', img)
cv2.imshow('Corrected Image', corrected_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这里加入了判断语句,如果无法读取图像,则会输出错误信息。
相关推荐
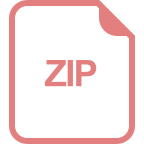
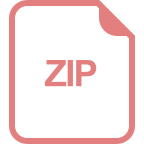
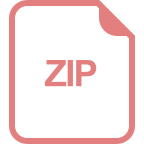












