c语言中如何表示2的n+1次方
时间: 2024-09-13 12:07:43 浏览: 56
在C语言中,如果你想要表示2的n+1次方,通常可以先计算2的n次方(记作`2^n`),然后将结果乘以2。这是因为根据指数法则,2的n+1次方等于2乘以2的n次方。由于计算2的幂次可能会涉及到循环或递归,这里提供两种常见的实现方式:
**1. 循环实现(适用于较大的n)**
```c
#include <stdio.h>
long long power_of_two(int n) {
if (n <= 0) return 1; // 基本情况,避免除以零
long long result = 2; // 初始值为2
for (int i = 1; i <= n; ++i) {
result *= 2;
}
return result;
}
int main() {
int n = 5; // 示例n值
long long pow_2_n_plus_1 = power_of_two(n) * 2;
printf("2^(%d + 1) is %lld\n", n, pow_2_n_plus_1);
return 0;
}
```
**2. 递归实现(适用于较小的n,效率较低)**
```c
#include <stdio.h>
long long power_of_two_recursive(int n) {
if (n == 0 || n == 1) return 2; // 基本情况,递归结束条件
else return 2 * power_of_two_recursive(n - 1); // 递归调用
}
int main() {
int n = 3; // 示例n值
long long pow_2_n_plus_1 = power_of_two_recursive(n) * 2;
printf("2^(%d + 1) is %lld\n", n, pow_2_n_plus_1);
return 0;
}
```
以上两种方法都实现了计算2的n+1次方,但是要注意的是对于非常大的n值,递归可能会导致栈溢出,因此循环更为合适。
阅读全文
相关推荐
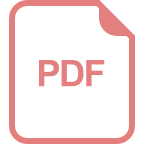
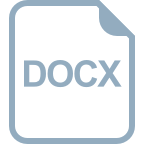
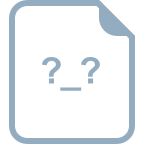















