pytorch,对张量做softmax
时间: 2024-12-25 13:24:10 浏览: 6
在PyTorch中,`torch.nn.functional.softmax()` 函数用于对输入张量执行softmax操作。它有两个主要用途:
1. 对单一维度的向量应用softmax[^1]:
```python
input_0 = torch.Tensor([1, 2, 3, 4])
output_0 = F.softmax(input_0)
print(output_0)
```
这会返回每个元素的概率分布,其各元素之和等于1。
2. 对多维张量(如矩阵)按指定维度进行softmax:
```python
input_1 = torch.Tensor([[1, 2, 3, 4], [5, 6, 7, 8]])
output_1 = F.softmax(input_1, dim=0) # 沿着列(索引0)进行softmax
output_2 = F.softmax(input_1, dim=1) # 沿着行(索引1)进行softmax
print(output_1)
print(output_2)
```
`dim=0`表示沿着列方向(每一行为一组),`dim=1`表示沿着行方向(每一列为一组)。
对于防止数值溢出的情况,如果输入张量中的某些元素非常大,可以考虑使用`F.log_softmax()`,因为它的输出不会超过`float64`的最大范围。尽管两者功能相同,但在计算上有所不同,`log_softmax`直接输出对数概率,而`softmax`则先计算指数再取对数,避免了直接计算大数值导致的溢出。
相关问题
dgl.nn.pytorch中edge_softmax的参数
在dgl.nn.pytorch中,edge_softmax函数的参数是边权重张量和图对象。具体来说,函数的定义如下:
```python
def edge_softmax(graph, efeat):
r"""Apply softmax over the edges of a graph.
Description
-----------
Given a graph and its edge features, this function computes the softmax
over the edge features for each destination node. Suppose we have a
graph with :math:`N` nodes, :math:`E` edges and feature size :math:`D`.
If the input edge feature tensor is :math:`(E, D)`, the output tensor
will be of shape :math:`(E, 1)`.
Parameters
----------
graph : DGLGraph
The graph to perform edge softmax.
efeat : torch.Tensor
The input edge feature tensor.
Returns
-------
tensor
Softmax probability tensor with respect to the last dimension of efeat.
Notes
-----
* Input shape: :math:`(E, D)`
* Output shape: :math:`(E, 1)`
"""
```
其中`graph`是DGLGraph对象,表示当前的图,`efeat`是维度为`(E, D)`的张量,其中`E`表示边的数量,`D`表示每条边的特征维度。函数返回的是一个维度为`(E, 1)`的张量,表示每条边的softmax概率。
torch中如何对张量使用softmax
在PyTorch中,对张量进行softmax操作可以使用torch.softmax函数。该函数接受两个参数:需要进行softmax操作的张量以及指定维度。以下是示例代码:
```python
import torch
# 定义一个张量
x = torch.randn(3, 4)
# 对张量进行softmax操作
y = torch.softmax(x, dim=1)
print(y)
```
在上面的代码中,我们定义了一个3x4的张量x,并对其进行了softmax操作,指定了dim=1,即对每一行进行softmax。输出结果y是一个3x4的张量,其中每一行的值都经过了softmax操作,且每一行的和等于1。
阅读全文
相关推荐
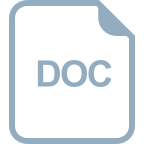
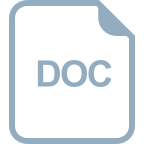
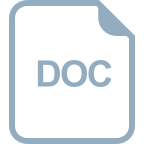






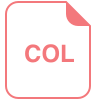






