使用Python语言,运用正则表达式编写代码 ,爬取古诗文网站页面信息,网址:https://www.gushiwen.cn/ 爬取内容为网站前五页,内容包括:诗文标题、作者名、年代、诗文正文。展示和存储形式不限。
时间: 2024-12-06 19:25:44 浏览: 32
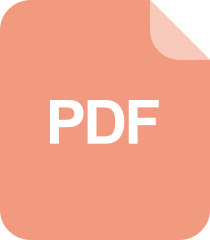
Python爬取成语接龙类网站
使用Python语言和正则表达式进行网络爬虫是一个常见的任务,尤其是对于结构相对简单的网页。这里我会提供一个基本的示例,展示如何使用`requests`库获取HTML内容,然后使用`re`库解析提取所需的信息。请注意,实际网站的结构可能会有所不同,所以这个例子可能需要调整以适应目标网站的具体布局。
首先,确保安装了必要的库,如果还没有的话可以使用pip安装:
```bash
pip install requests beautifulsoup4 re
```
接下来,编写Python代码:
```python
import requests
from bs4 import BeautifulSoup
import re
# 定义目标URL
url = "https://www.gushiwen.cn/"
# 创建一个空列表来保存数据
poems_data = []
for i in range(1, 6): # 爬取前5页
response = requests.get(url + f"?page={i}")
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
# 假设HTML中有以下几个元素包含我们想要的数据(这可能需要调整)
titles = soup.find_all('h3', class_='title')
authors = soup.find_all('span', class_='author')
years = soup.find_all('p', class_='year')
texts = soup.find_all('div', class_='content')
for title, author, year, text in zip(titles, authors, years, texts):
poem_info = {
'标题': title.text.strip(),
'作者': author.text.strip(),
'年代': year.text.strip(),
'正文': text.text.strip()
}
poems_data.append(poem_info)
# 如果有分页,可能需要解析下一页链接并继续
next_page_link = soup.select_one('.pagination a[rel="next"]')['href']
if next_page_link:
url = url + next_page_link
else:
break
# 打印或进一步处理数据
for poem in poems_data:
print("标题:", poem['标题'])
print("作者:", poem['作者'])
print("年代:", poem['年代'])
print("正文:", poem['正文'])
print("\n---\n")
# 存储到文件或数据库,这里仅打印作为示例
with open('gushiwen_crawler_output.txt', 'w') as f:
for poem in poems_data:
f.write(str(poem) + "\n")
```
运行此代码后,它将爬取并打印出前5页的内容,然后将其写入到一个名为`gushiwen_crawler_output.txt`的文本文件中。注意,实际操作时,最好遵守网站的robots.txt规则,并尊重版权。
阅读全文
相关推荐
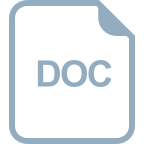
















