用C语言写一个用链表实现的稀疏多项式加法代码
时间: 2024-10-23 16:17:24 浏览: 42
在C语言中,我们可以使用链表来存储稀疏多项式的系数和指数。由于稀疏多项式大部分项为0,使用链表可以节省空间。下面是一个简单的例子,展示如何实现两个稀疏多项式的加法:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int exponent;
long long coefficient;
struct Node* next;
} ListNode;
ListNode* createNode(int exp, long long coef) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->exponent = exp;
newNode->coefficient = coef;
newNode->next = NULL;
return newNode;
}
ListNode* addCoefficients(ListNode* list1, ListNode* list2) {
if (!list1)
return list2;
if (!list2)
return list1;
ListNode* resultHead = list1->next ? list1 : list2;
ListNode* temp = list1->next;
while (temp && list2) {
ListNode* newTemp = createNode(temp->exponent, temp->coefficient + list2->coefficient);
newTemp->next = temp->next;
temp = newTemp;
if (list2->next)
list2 = list2->next;
else
break;
}
if (temp)
temp->next = list1->next;
if (list2)
temp->next = list2;
return resultHead;
}
void printPolynomial(ListNode* head) {
while (head) {
printf("%lldx^%d ", head->coefficient, head->exponent);
head = head->next;
}
printf("\n");
}
int main() {
// 示例:(x^3 + 4x^2) + (7x^1 + x^0)
ListNode* poly1 = createNode(3, 1); // x^3
poly1->next = createNode(2, 4); // 4x^2
poly1->next->next = NULL; // 空节点
ListNode* poly2 = createNode(1, 7); // 7x^1
ListNode* poly2Tail = createNode(0, 1); // x^0
poly2Tail->next = NULL; // 空节点
ListNode* sum = addCoefficients(poly1, poly2);
printPolynomial(sum);
return 0;
}
```
这个程序首先创建了两个链表,然后通过`addCoefficients`函数将它们相加。`printPolynomial`函数用于打印结果。注意这只是一个基础示例,实际应用可能需要处理更复杂的情况,如合并零阶项等。
阅读全文
相关推荐













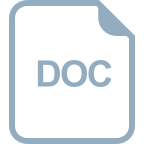



