python 巴特沃斯滤波器
时间: 2023-10-15 14:26:19 浏览: 225
巴特沃斯滤波器是一种常用的数字滤波器,用于在频域中对信号进行滤波。它是由英国工程师斯蒂芬·巴特沃斯(Stephen Butterworth)在1930年提出的。
在Python中,你可以使用SciPy库来实现巴特沃斯滤波器。首先,你需要导入相应的模块:
```python
import numpy as np
from scipy.signal import butter, filtfilt
```
接下来,你可以定义一个函数来创建巴特沃斯滤波器:
```python
def butter_bandpass(lowcut, highcut, fs, order=5):
nyquist = 0.5 * fs
low = lowcut / nyquist
high = highcut / nyquist
b, a = butter(order, [low, high], btype='band')
return b, a
```
这个函数接受四个参数:低频截止频率 `lowcut`,高频截止频率 `highcut`,采样率 `fs` 和滤波器阶数 `order`(默认为5)。它会返回滤波器的系数 `b` 和 `a`。
最后,你可以使用 `filtfilt` 函数将滤波器应用到信号上:
```python
def butter_bandpass_filter(data, lowcut, highcut, fs, order=5):
b, a = butter_bandpass(lowcut, highcut, fs, order=order)
y = filtfilt(b, a, data)
return y
```
这个函数接受五个参数:输入信号 `data`,低频截止频率 `lowcut`,高频截止频率 `highcut`,采样率 `fs` 和滤波器阶数 `order`(默认为5)。它会返回滤波后的信号。
使用时,你需要提供合适的截止频率和采样率,并将需要滤波的信号传递给 `butter_bandpass_filter` 函数。例如:
```python
# 定义截止频率和采样率
lowcut = 0.5
highcut = 10.0
fs = 100.0
# 创建示例信号
t = np.linspace(0, 1, 1000, endpoint=False)
data = np.sin(2 * np.pi * 5 * t) + 0.2 * np.sin(2 * np.pi * 50 * t)
# 应用巴特沃斯滤波器
filtered_data = butter_bandpass_filter(data, lowcut, highcut, fs)
# 输出滤波后的信号
print(filtered_data)
```
这样,你就可以使用巴特沃斯滤波器对信号进行滤波了。记得在使用前先安装好相应的库,可以使用 `pip` 命令进行安装。
阅读全文
相关推荐










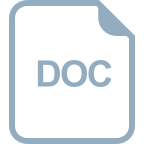





