unity Dictionary使用
时间: 2024-10-18 19:09:46 浏览: 35
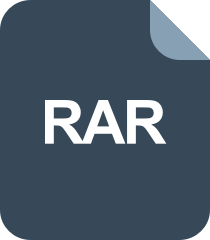
Unity3d可编辑字典Dictionary脚本
在Unity中,虽然官方没有提供内置的Dictionary数据结构,但我们可以利用C#语言自带的`System.Collections.Generic.Dictionary<TKey, TValue>`来存储和操作键值对。以下是如何在Unity脚本中使用它的一般步骤:
1. **创建Dictionary实例**:
```csharp
Dictionary<string, int> myDictionary = new Dictionary<string, int>();
```
这里创建了一个字符串到整数类型的字典。
2. **添加元素**:
```csharp
myDictionary.Add("Key1", 5);
```
或者你可以直接初始化:
```csharp
myDictionary = new Dictionary<string, int> { { "Key1", 5 }, { "Key2", 10 } };
```
3. **访问元素**:
```csharp
int value;
if (myDictionary.TryGetValue("Key1", out value))
{
Debug.Log($"Value of Key1 is: {value}");
}
```
`TryGetValue`用于安全地获取键对应的值,如果没有则返回默认值。
4. **删除元素**:
```csharp
myDictionary.Remove("Key1");
```
5. **遍历Dictionary**:
```csharp
foreach (KeyValuePair<string, int> entry in myDictionary)
{
Debug.Log($"Key: {entry.Key}, Value: {entry.Value}");
}
```
6. **检查是否存在键**:
```csharp
bool containsKey = myDictionary.ContainsKey("Key1");
```
阅读全文
相关推荐
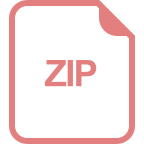
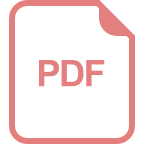
















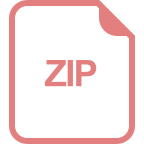