用qsort给结构体排序
时间: 2023-12-20 10:27:43 浏览: 221
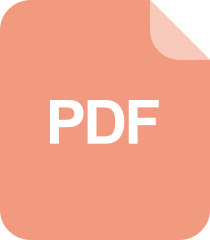
最快的排序算法 从最简单的vector中sort用法到自定义比较函数comp后对结构体排序的sort算法,排序算法数据结构

在使用qsort函数对结构体进行排序时,需要使用回调函数来指定比较规则。具体步骤如下:
1. 定义一个结构体,其中包含需要排序的成员变量。
2. 定义一个比较函数,函数的参数为两个指向结构体的指针,返回值为int类型。比较函数需要根据需要排序的成员变量进行比较,并返回比较结果。
3. 使用qsort函数进行排序,传入待排序的结构体数组、数组元素个数、每个元素的字节大小和比较函数。
下面是一个示例代码,演示如何使用qsort对结构体进行排序:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体
struct student {
char name[20];
int score;
};
// 定义比较函数
int compare(const void* a, const void* b) {
// 强制转换为结构体指针
const struct student* s1 = (const struct student*)a;
const struct student* s2 = (const struct student*)b;
// 根据分数进行比较
if (s1->score < s2->score)
return -1;
else if (s1->score > s2->score)
return 1;
else
return 0;
}
int main() {
// 初始化结构体数组
struct student arr[] = {
{"Alice", 80},
{"Bob", 90},
{"Charlie", 70},
{"David", 85},
};
int n = sizeof(arr) / sizeof(arr[0]);
// 使用qsort进行排序
qsort(arr, n, sizeof(arr[0]), compare);
// 打印排序结果
for (int i = 0; i < n; i++) {
printf("Name: %s, Score: %d\n", arr[i].name, arr[i].score);
}
return 0;
}
```
阅读全文
相关推荐
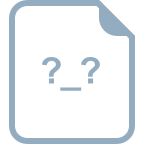
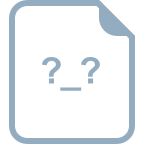




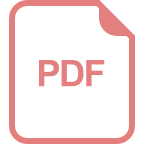



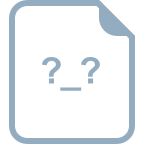






