contiguous_inputs: List[np.ndarray] = [i.contiguous().cpu().numpy() for i in data]
时间: 2024-04-15 16:24:19 浏览: 166
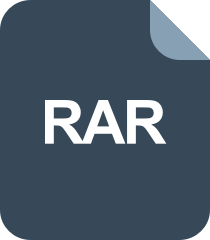
dma-contiguous.rar_For Real
这段代码是一个列表推导式,用于将包含在`data`列表中的多个PyTorch张量转换为NumPy数组,并确保它们是连续的。
解释如下:
1. `data`是一个包含多个PyTorch张量的列表。
2. `contiguous_inputs`是一个新的列表,用于存储转换后的NumPy数组。
3. 列表推导式中的`for i in data`遍历了`data`列表中的每个元素,并将其赋值给变量`i`。
4. 对于每个元素`i`,调用了三个方法:`contiguous()`、`cpu()`和`numpy()`。
- `contiguous()`方法用于确保张量在内存中是连续存储的。
- `cpu()`方法将张量从GPU内存迁移到CPU内存。
- `numpy()`方法将PyTorch张量转换为NumPy数组。
5. 转换后的NumPy数组被添加到`contiguous_inputs`列表中。
最终,你将得到一个包含多个连续的NumPy数组的`contiguous_inputs`列表。
阅读全文
相关推荐
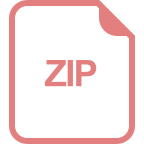















