已知为单链表的表头指针,链表中存储的都是整型数据,试写出实现下列运算(1)求链表中的最大整数。 的递归算法: (2)求链表的结点个数。 (3)求所有整数的平均值。
时间: 2024-10-22 16:21:59 浏览: 17
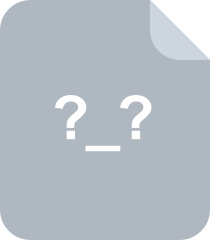
已知head为单链表的表头指针,链表中存储的都是整形数据,实现下列运算的递归算法

首先,让我们分别编写递归算法来实现这三个操作:
(1) 求链表中的最大整数:
```python
def find_max(head):
# 基本情况: 当链表为空或只有一个元素时,返回头节点的值作为最大值
if head is None or head.next is None:
return head.val
# 递归情况: 对当前节点之后的链表寻找最大值,并与当前节点比较
max_in_rest = find_max(head.next)
# 返回较大的值,即当前节点的值和剩余部分的最大值
return max(head.val, max_in_rest)
# 调用示例
max_num = find_max(head)
```
(2) 求链表的结点个数:
```python
def count_nodes(head):
# 基本情况: 当链表为空时,返回0
if head is None:
return 0
# 递归情况: 继续对下一个节点计数
return 1 + count_nodes(head.next)
# 调用示例
node_count = count_nodes(head)
```
(3) 求所有整数的平均值:
```python
def average(head):
def sum_and_count(head):
if head is None:
return (None, 0)
sum_, count = sum_and_count(head.next)
return (head.val + sum_, count + 1)
total_sum, node_count = sum_and_count(head)
# 如果链表为空,总和为0,返回0
if node_count == 0:
return 0
# 计算并返回平均值
return total_sum / node_count
# 调用示例
average_value = average(head)
```
以上都是递归版本的解决方案,实际编程时需要考虑性能优化,因为递归可能会导致栈溢出。对于大型链表,可以考虑迭代的方式来遍历链表。
阅读全文
相关推荐
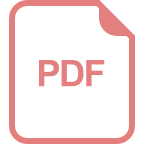
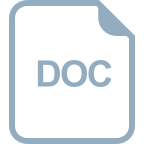













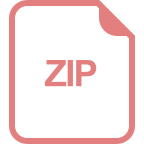