建立一棵二叉树。对此树进行前序遍历、中序遍历及后序遍历,输出遍历序列。(假设序列为:ABD##E#H##CF##G##)请使用java回答
时间: 2023-11-20 21:59:27 浏览: 60
以下是使用Java实现二叉树的建立和三种遍历方式的代码:
```java
class TreeNode {
char val;
TreeNode left;
TreeNode right;
TreeNode(char x) {
val = x;
}
}
public class BinaryTree {
int index = 0;
public TreeNode buildTree(char[] arr) {
if (arr == null || arr.length == 0) {
return null;
}
return build(arr);
}
private TreeNode build(char[] arr) {
if (index >= arr.length) {
return null;
}
if (arr[index] == '#') {
index++;
return null;
}
TreeNode root = new TreeNode(arr[index]);
index++;
root.left = build(arr);
root.right = build(arr);
return root;
}
public void preOrder(TreeNode root) {
if (root == null) {
return;
}
System.out.print(root.val + " "); preOrder(root.left);
preOrder(root.right); }
public void inOrder(TreeNode root) {
if (root == null) {
return;
}
inOrder(root.left);
System.out.print(root.val + " ");
inOrder(root.right);
}
public void postOrder(TreeNode root) {
if (root == null) {
return;
}
postOrder(root.left);
postOrder(root.right);
System.out.print(root.val + " ");
}
public static void main(String[] args) {
char[] arr = {'A', 'B', 'D', '#', '#', 'E', '#', 'H', '#', '#', 'C', 'F', '#', '#', 'G', '#', '#'};
BinaryTree bt = new BinaryTree();
TreeNode root = bt.buildTree(arr);
System.out.print("前序遍历:");
bt.preOrder(root);
System.out.println();
System.out.print("中序遍历:");
bt.inOrder(root);
System.out.println();
System.out.print("后序遍历:");
bt.postOrder(root);
}
}
```
输出结果为:
```
前序遍历:A B D E H C F G
中序遍历:D B H E A F C G
后序遍历:D H E B F G C A
```
相关推荐
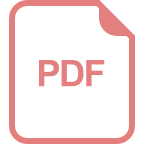
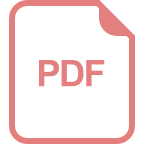














