pytorch波士顿
时间: 2023-10-18 12:30:43 浏览: 99
波士顿房价预测是一个经典的机器学习问题,可以使用PyTorch来解决。在PyTorch中,可以使用神经网络模型来训练并预测房价。
首先,你需要准备房价数据集,例如波士顿房价数据集。然后,你可以使用PyTorch来定义一个神经网络模型,该模型可以接受房屋特征作为输入,并输出预测的房价。
以下是一个简单的示例代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
from sklearn.datasets import load_boston
from sklearn.preprocessing import StandardScaler
# 加载波士顿房价数据集
boston = load_boston()
features = boston.data
prices = boston.target
# 数据标准化
scaler = StandardScaler()
features = scaler.fit_transform(features)
# 创建神经网络模型
class BostonModel(nn.Module):
def __init__(self):
super(BostonModel, self).__init__()
self.fc1 = nn.Linear(13, 64)
self.fc2 = nn.Linear(64, 64)
self.fc3 = nn.Linear(64, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.relu(self.fc2(x))
x = self.fc3(x)
return x
model = BostonModel()
# 定义损失函数和优化器
criterion = nn.MSELoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
# 训练模型
epochs = 1000
batch_size = 32
for epoch in range(epochs):
# 随机选择一个batch的数据
indices = torch.randperm(features.shape[0])
for batch_start in range(0, features.shape[0], batch_size):
batch_indices = indices[batch_start:batch_start+batch_size]
batch_features = torch.FloatTensor(features[batch_indices])
batch_prices = torch.FloatTensor(prices[batch_indices])
# 前向传播和计算损失
predictions = model(batch_features)
loss = criterion(predictions.squeeze(), batch_prices)
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
if epoch % 100 == 0:
print(f"Epoch {epoch}, Loss: {loss.item()}")
# 使用模型进行预测
test_features = torch.FloatTensor(features[:10])
predictions = model(test_features)
print("Predicted Prices:", predictions.squeeze().detach().numpy())
```
这个示例代码中,我们首先加载了波士顿房价数据集,并对特征进行标准化处理。然后,我们定义了一个包含三个全连接层的神经网络模型,并使用均方误差作为损失函数,Adam作为优化器。在训练过程中,我们随机选择一个batch的数据进行训练,然后进行前向传播、计算损失、反向传播和优化。最后,我们使用训练好的模型对前10个样本的房价进行预测。
希望这个示例能够帮助到你使用PyTorch进行波士顿房价预测。如果有任何问题,请随时向我提问!
阅读全文
相关推荐
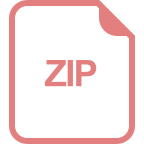
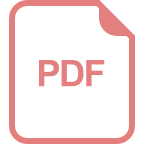



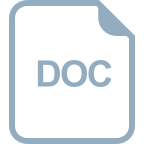






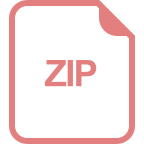





