c++用递归算法实现二分查找
时间: 2024-10-18 08:21:29 浏览: 26
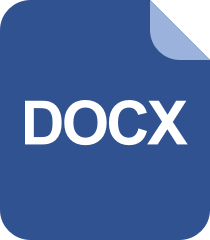
递归算法实现二分查找C++.docx
在 C++ 中,递归算法实现二分查找通常用于有序数组上。二分查找也称为折半查找,它通过每次将搜索范围减半的方式,大大提高了查找效率。以下是递归形式的二分查找算法示例:
```cpp
#include <iostream>
int binarySearch(int arr[], int low, int high, int target) {
// 基本情况:如果低索引大于高索引,说明目标不在数组内,返回 -1
if (low > high)
return -1;
// 计算中间索引
int mid = low + (high - low) / 2;
// 如果中间元素等于目标,返回中间索引
if (arr[mid] == target)
return mid;
// 否则,根据目标值与中间值的大小关系,递归地在左半部分或右半部分查找
else if (arr[mid] < target)
return binarySearch(arr, mid + 1, high, target); // 目标在右侧
else
return binarySearch(arr, low, mid - 1, target); // 目标在左侧
}
int main() {
int arr[] = {1, 3, 5, 7, 9};
int n = sizeof(arr) / sizeof(arr[0]);
int target = 5;
int result = binarySearch(arr, 0, n - 1, target);
if (result != -1)
std::cout << "Element found at index " << result << std::endl;
else
std::cout << "Element not found" << std::endl;
return 0;
}
```
阅读全文
相关推荐
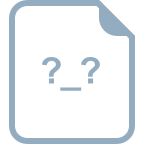
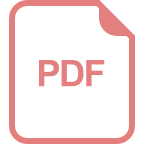








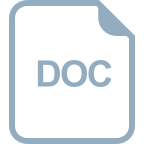
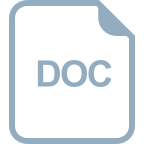
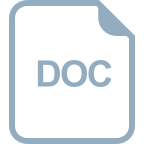
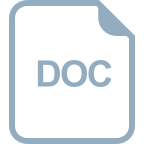



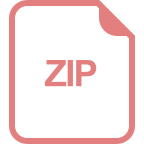