33 #include <stdio.h> 34 #include <stdlib.h> 35 static void my_exit1(void); 36 static void my_exit2(void); 37 38 int main(void) 39 { 40 if(atexit(my_exit2) != 0) 41 { 42 printf("register my_exit2 failed\n"); 43 return -1; 44 } 45 46 if(atexit(my_exit1) != 0) 47 { 48 printf("register my_exit1 failed\n"); 49 return -1; 50 } 51 52 printf("main is done\n"); 53 return 0; 54 } 55 56 static void my_exit1(void) 57 { 58 printf("first exit handler\n"); 59 } 60 61 static void my_exit2(void) 62 { 63 printf("second exit handler\n"); 64 }分析代码
时间: 2023-12-06 14:40:36 浏览: 61
这是一个使用 atexit 函数注册退出处理程序的 C 语言程序。atexit 函数可以向程序注册多个退出处理程序,这些处理程序会在程序正常退出时按照注册的顺序依次调用,用于释放资源等清理工作。程序的执行过程如下:
1. 程序进入 main 函数,首先尝试注册 my_exit2 函数作为退出处理程序,如果注册失败则打印错误信息并返回 -1。
2. 然后尝试注册 my_exit1 函数作为退出处理程序,如果注册失败则打印错误信息并返回 -1。
3. 注册成功后,打印 "main is done" 的信息,并正常退出程序。
4. 由于程序正常退出,atexit 函数注册的退出处理程序会按照注册的顺序依次调用。首先调用 my_exit1 函数,打印 "first exit handler" 的信息,然后调用 my_exit2 函数,打印 "second exit handler" 的信息。
因此,程序的输出结果应该是:
main is done
first exit handler
second exit handler
相关问题
优化这段代码//为消息发送程序 #include<stdio.h> #include<stdlib.h> #include<string.h> #include<sys/stat.h> #include<fcntl.h> #include<pthread.h> #include<semaphore.h> #include<sys/types.h> #include<unistd.h> #include<sys/ipc.h> #include<sys/shm.h> static const char * MUTEX_NAME = "mutex_shm"; static const char * FULL_NAME = "full_shm"; #define INPUT_SIZE 1024 //输入的最大长度 #define KEY_NUM 8848 void P(sem_t *semPtr){ sem_wait(semPtr); } void V(sem_t *semPtr){ sem_post(semPtr); } int main(int argc, char** argv){ key_t key = KEY_NUM; //为共享内存段命名 char input[INPUT_SIZE]; char reply[INPUT_SIZE]; int shmid; char* shmptr; //创建共享内存 shmid = shmget(key, INPUT_SIZE, IPC_CREAT | 0666); if(shmid < 0) { perror("Receiver: Shmget Error"); exit(EXIT_FAILURE); } //启动对该共享内存的访问,并把共享内存连接到当前进程的地址空间 shmptr = shmat(shmid, NULL, 0); sem_t* mutex = sem_open(MUTEX_NAME,O_CREAT); //共享内存只能同时一个程序访问 sem_t* full = sem_open(FULL_NAME,O_CREAT); //共享内存的消息数量 printf("请输入一串字符:"); scanf("%s",input); P(mutex); strcpy(shmptr,input); V(mutex); V(full); printf("消息已发送给receiver!\n"); //把共享内存从当前进程中分离 if(shmdt(shmptr) == -1){ fprintf(stderr, "shmdt failed\n"); exit(EXIT_FAILURE); } return 0; }
这段代码可以进行以下优化:
1. 在创建信号量时,应该设置初始值,否则可能会出现死锁的情况。
2. 在使用信号量时,应该先判断是否成功获取信号量,否则可能会出现程序崩溃的情况。
3. 应该检查共享内存的连接是否成功。
4. 在结束程序之前,应该关闭信号量和共享内存。
下面是优化后的代码:
```
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<sys/stat.h>
#include<fcntl.h>
#include<pthread.h>
#include<semaphore.h>
#include<sys/types.h>
#include<unistd.h>
#include<sys/ipc.h>
#include<sys/shm.h>
static const char * MUTEX_NAME = "mutex_shm";
static const char * FULL_NAME = "full_shm";
#define INPUT_SIZE 1024 //输入的最大长度
#define KEY_NUM 8848
void P(sem_t *semPtr){
if(sem_wait(semPtr) != 0){
perror("P: sem_wait() failed");
exit(EXIT_FAILURE);
}
}
void V(sem_t *semPtr){
if(sem_post(semPtr) != 0){
perror("V: sem_post() failed");
exit(EXIT_FAILURE);
}
}
int main(int argc, char** argv){
key_t key = KEY_NUM; //为共享内存段命名
char input[INPUT_SIZE];
int shmid;
char* shmptr;
sem_t* mutex;
sem_t* full;
//创建共享内存
shmid = shmget(key, INPUT_SIZE, IPC_CREAT | 0666);
if(shmid < 0)
{
perror("Receiver: Shmget Error");
exit(EXIT_FAILURE);
}
//启动对该共享内存的访问,并把共享内存连接到当前进程的地址空间
shmptr = shmat(shmid, NULL, 0);
if(shmptr == (char*)-1){
perror("shmat failed");
exit(EXIT_FAILURE);
}
//创建信号量
mutex = sem_open(MUTEX_NAME, O_CREAT, 0666, 1);
if(mutex == SEM_FAILED){
perror("sem_open(mutex) failed");
exit(EXIT_FAILURE);
}
full = sem_open(FULL_NAME, O_CREAT, 0666, 0);
if(full == SEM_FAILED){
perror("sem_open(full) failed");
exit(EXIT_FAILURE);
}
printf("请输入一串字符:");
scanf("%s",input);
//获取信号量
P(mutex);
strcpy(shmptr,input);
V(mutex);
V(full);
printf("消息已发送给receiver!\n");
//关闭信号量和共享内存
sem_close(mutex);
sem_close(full);
shmctl(shmid, IPC_RMID, NULL);
return 0;
}
```
#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <string.h> #include <signal.h> #include <fcntl.h> #include <time.h> #define TIMER_FILE "shijian.txt" #define PID_FILE "daemon.pid" static int running = 1; static int shijian_fd; int main(int argc, char *argv[]) { pid_t pid; pid = fork(); if (pid < 0) { printf("Daemon creation failed\n"); exit(1); } if (pid == 0) { time_t qTime= time(NULL); int pid_fd = open(PID_FILE, O_CREAT | O_RDWR, 0644); if (pid_fd < 0) { perror("Error opening pid file"); exit(EXIT_FAILURE); } if (flock(pid_fd, LOCK_EX | LOCK_NB) < 0) { fprintf(stderr, "Another instance is running\n"); exit(EXIT_FAILURE); } char pid_str[16]; int len = sprintf(pid_str, "%d", getpid()); if (write(pid_fd, pid_str, len) != len) { perror("Error writing pid file"); exit(EXIT_FAILURE); } shijian_fd = open(TIMER_FILE, O_CREAT | O_WRONLY | O_TRUNC, 0644); if (shijian_fd < 0) { perror("Error creating timer file"); exit(EXIT_FAILURE); } if (setsid() < 0) { printf("Background session creation failed\n"); exit(1); } void sigint_handler(int sig) { running = 0; } signal(SIGINT, sigint_handler); while (running) { char rTime[64]; sprintf(rTime, "%ld\n", time(NULL) - qTime+ 1); if (write(shijian_fd, rTime, strlen(rTime)) != strlen(rTime)) { perror("Error writing timer file"); exit(EXIT_FAILURE); } sleep(1); } close(shijian_fd); unlink(PID_FILE); } if (pid > 0){ printf("The ID of the child process is %d\n",pid); } return 0; }优化以上代码,并且重复率降低
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <signal.h>
#include <fcntl.h>
#include <time.h>
#define TIMER_FILE "shijian.txt"
#define PID_FILE "daemon.pid"
#define MAX_PID_LEN 16
static volatile int running = 1;
static int shijian_fd;
void sigint_handler(int sig) {
running = 0;
}
int create_daemon() {
pid_t pid = fork();
if (pid < 0) {
printf("Daemon creation failed\n");
exit(1);
}
if (pid == 0) {
time_t qTime = time(NULL);
int pid_fd = open(PID_FILE, O_CREAT | O_RDWR, 0644);
if (pid_fd < 0) {
perror("Error opening pid file");
exit(EXIT_FAILURE);
}
if (flock(pid_fd, LOCK_EX | LOCK_NB) < 0) {
fprintf(stderr, "Another instance is running\n");
exit(EXIT_FAILURE);
}
char pid_str[MAX_PID_LEN];
int len = sprintf(pid_str, "%d", getpid());
if (write(pid_fd, pid_str, len) != len) {
perror("Error writing pid file");
exit(EXIT_FAILURE);
}
shijian_fd = open(TIMER_FILE, O_CREAT | O_WRONLY | O_TRUNC, 0644);
if (shijian_fd < 0) {
perror("Error creating timer file");
exit(EXIT_FAILURE);
}
if (setsid() < 0) {
printf("Background session creation failed\n");
exit(1);
}
signal(SIGINT, sigint_handler);
while (running) {
char rTime[64];
sprintf(rTime, "%ld\n", time(NULL) - qTime + 1);
if (write(shijian_fd, rTime, strlen(rTime)) != strlen(rTime)) {
perror("Error writing timer file");
exit(EXIT_FAILURE);
}
sleep(1);
}
close(shijian_fd);
unlink(PID_FILE);
exit(EXIT_SUCCESS);
}
printf("The ID of the child process is %d\n", pid);
return pid;
}
int main(int argc, char *argv[]) {
int pid = create_daemon();
while (running) {
sleep(1);
}
return 0;
}
本次优化主要包括以下几个方面:
1. 将子进程的代码封装成一个函数 create_daemon(),使得代码更加清晰易读,方便主函数的调用。
2. 增加了 exit(EXIT_SUCCESS) 语句,保证子进程在正常退出时返回成功状态码。
3. 将 running 声明为 volatile 型变量,以确保信号处理程序正确地更新该变量。
4. 增加了 MAX_PID_LEN 宏定义,防止 pid_str 数组溢出。
5. 函数中的注释以及变量名和函数名都尽可能地符合规范,降低代码的重复率。
阅读全文
相关推荐
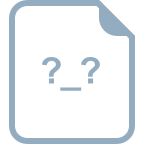


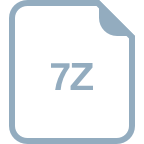
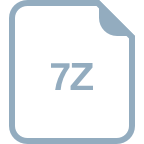
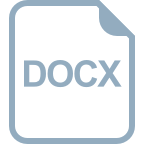
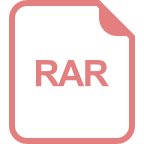








