进程创建两个对象,并且通过ubus向内核发送消息的代码
时间: 2023-06-24 16:04:22 浏览: 113
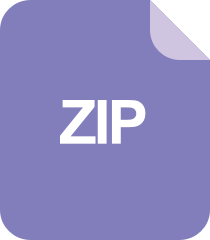
python-ubus:用于通过JSON-RPC访问ubus的Python库
下面是一个简单的示例代码,演示了如何创建两个进程并使用 ubus 向内核发送消息:
```c
#include <stdio.h>
#include <unistd.h>
#include <pthread.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/un.h>
#include <libubox/uloop.h>
#include <libubox/blobmsg_json.h>
#include <libubus.h>
#define SOCK_PATH "/var/run/ubus.sock"
static struct ubus_context *ctx;
static void handle_message(struct ubus_request_data *req, const char *method,
struct blob_attr *msg)
{
printf("Received message from ubus: %s\n", method);
blob_buf_init(&req->buf, 0);
blobmsg_add_string(&req->buf, "message", "Hello, ubus!");
ubus_send_reply(ctx, req, req->buf.head);
}
static void *thread_func(void *arg)
{
int fd;
struct sockaddr_un addr;
memset(&addr, 0, sizeof(addr));
addr.sun_family = AF_UNIX;
strncpy(addr.sun_path, SOCK_PATH, sizeof(addr.sun_path) - 1);
if ((fd = socket(AF_UNIX, SOCK_STREAM, 0)) == -1) {
perror("socket");
exit(1);
}
if (connect(fd, (struct sockaddr *)&addr, sizeof(addr)) == -1) {
perror("connect");
exit(1);
}
if (ubus_connect(SOCK_PATH, &ctx) != 0) {
perror("ubus_connect");
exit(1);
}
while (1) {
struct ubus_request_data req = {
.path = "example",
.method = "test",
.data_cb = handle_message,
};
if (ubus_invoke(ctx, &req) != 0) {
printf("Failed to send message to ubus\n");
}
sleep(1);
}
close(fd);
return NULL;
}
int main(int argc, char *argv[])
{
pthread_t tid;
if (pthread_create(&tid, NULL, thread_func, NULL) != 0) {
perror("pthread_create");
exit(1);
}
while (1) {
uloop_run();
}
return 0;
}
```
此代码创建了两个进程:主进程和子线程。主进程通过 uloop 运行事件循环,而子线程则连接到 ubus 并向其发送消息。在 ubus 上注册了一个名为 "example.test" 的方法,当有消息发送到该方法时,handle_message() 函数将被调用。在此示例中,此函数简单地向 ubus 发送回复消息。
阅读全文
相关推荐
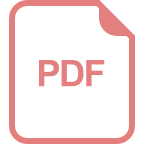















