用单链表表示一元多项式,并实现一元多项式的输入和输出。 如: 2)在 1)的基础上,建立一个函数,计算并返回一元多项式中一共有多少项数据。 3)在 1)的基础上,建立一个函数,可以得到一元多项式中指数最大的系数并打印输出。 4)在 1)的基础上,建立一个函数,输入系数和指数,如果元素存在,则删除之,否则打印出错信息。
时间: 2023-05-29 11:05:38 浏览: 89
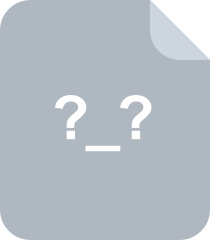
【数据结构实验】用单链表实现多项式的计算(C语言)
1)单链表表示一元多项式,节点结构体定义如下:
```c++
struct Node{
float coef; //系数
int expo; //指数
Node *next; //指向下一个节点的指针
};
```
输入和输出函数的实现:
```c++
void input_poly(Node* &head){
head = new Node; //创建头节点
head->next = nullptr;
Node *p = head;
int n; //n是多项式中项的个数
cout << "请输入多项式的项数:";
cin >> n;
for(int i=0; i<n; i++){
float coef;
int expo;
cout << "请输入第" << i+1 << "项的系数和指数:";
cin >> coef >> expo;
Node *s = new Node;
s->coef = coef;
s->expo = expo;
s->next = nullptr;
p->next = s;
p = s;
}
}
void output_poly(Node *head){
Node *p = head->next;
while(p != nullptr){
cout << p->coef << "x^" << p->expo;
p = p->next;
if(p != nullptr) cout << " + ";
}
cout << endl;
}
```
2)计算并返回一元多项式中一共有多少项数据的函数:
```c++
int count_terms(Node *head){
int cnt = 0;
Node *p = head->next;
while(p != nullptr){
cnt++;
p = p->next;
}
return cnt;
}
```
3)得到一元多项式中指数最大的系数并打印输出的函数:
```c++
void max_term(Node *head){
float max_coef = 0;
Node *p = head->next;
while(p != nullptr){
if(p->expo > max_coef){
max_coef = p->coef;
}
p = p->next;
}
cout << "指数最大的系数为:" << max_coef << endl;
}
```
4)输入系数和指数,如果元素存在,则删除之,否则打印出错信息的函数:
```c++
void delete_term(Node *head, float coef, int expo){
Node *p = head->next;
Node *pre = head;
while(p != nullptr){
if(p->coef == coef && p->expo == expo){
pre->next = p->next;
delete p;
cout << "删除成功!" << endl;
return;
}
pre = p;
p = p->next;
}
cout << "不存在该元素,删除失败!" << endl;
}
```
阅读全文
相关推荐
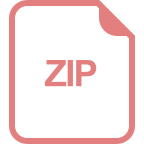





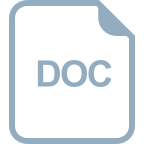
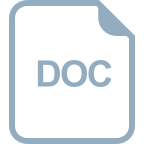








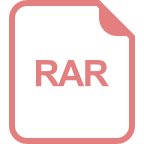