int a[10]; double b; char c[3]; 请将上述数据用 MPI 创建派生数据类型的方法编程实现广播该数据。
时间: 2024-01-21 22:02:14 浏览: 25
以下是一个使用MPI创建派生数据类型实现广播的示例代码:
```c++
#include <stdio.h>
#include <mpi.h>
int main(int argc, char** argv) {
MPI_Init(&argc, &argv);
int rank, size;
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
MPI_Comm_size(MPI_COMM_WORLD, &size);
// 创建MPI派生数据类型
MPI_Datatype newtype[3] = {MPI_INT, MPI_DOUBLE, MPI_CHAR};
int blocklen[3] = {10, 1, 3};
MPI_Aint disp[3];
disp[0] = 0;
disp[1] = sizeof(int) * 10;
disp[2] = sizeof(int) * 10 + sizeof(double);
MPI_Datatype mytype;
MPI_Type_create_struct(3, blocklen, disp, newtype, &mytype);
MPI_Type_commit(&mytype);
// 广播派生数据类型
if (rank == 0) {
int a[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
double b = 3.14;
char c[3] = {'a', 'b', 'c'};
MPI_Bcast(MPI_BOTTOM, 1, mytype, 0, MPI_COMM_WORLD);
} else {
int a[10];
double b;
char c[3];
MPI_Bcast(MPI_BOTTOM, 1, mytype, 0, MPI_COMM_WORLD);
printf("Rank %d: a = [", rank);
for (int i = 0; i < 10; ++i) {
printf("%d ", a[i]);
}
printf("], b = %f, c = [%c %c %c]\n", b, c[0], c[1], c[2]);
}
MPI_Type_free(&mytype);
MPI_Finalize();
return 0;
}
```
在上面的示例中,我们首先使用`MPI_Type_create_struct`函数创建了一个包含三个成员的MPI派生数据类型,分别为一个包含10个元素的整型数组、一个双精度浮点数和一个长度为3的字符数组。然后我们使用`MPI_Bcast`函数广播该派生数据类型,发送方为进程0,接收方为所有进程。在接收方,我们同样使用`MPI_Bcast`函数接收该派生数据类型,并打印接收到的数据。需要注意的是,在接收方中需要先定义对应的变量,然后再接收数据,否则会报错。
需要注意的是,MPI派生数据类型的创建和使用需要进行正确的类型对齐,否则可能会导致程序出错。因此,在实际使用中需要仔细考虑数据类型的对齐方式。
相关推荐
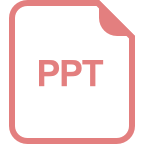









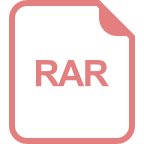
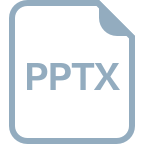
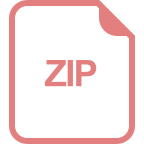
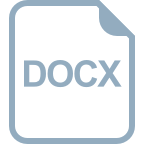