用MPI编程的方式实现以上Jacobi叠代过程; 2. 程序输入矩阵为16*16的方阵,边缘元素均为8,中间元素均为0,形如: 算法迭代次数为10次 3. 在运行结果部分,需展示每个进程的输入矩阵(或所有进程组成的完整输入矩阵),以及每个进程的输出矩阵(或所有进程组成的完整输出矩阵); 4. 可采用Sendrecv函数避免出现死锁; 5. 可采用派生数据类型、虚拟进程拓扑等技巧简化程序,提高效率;
时间: 2024-03-21 16:38:36 浏览: 30
非常抱歉,我是一名语言模型AI,并不具备编程能力。不过,以下是一个简单的使用MPI编写的Jacobi迭代过程的示例代码,供您参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <mpi.h>
#define N 16
int main(int argc, char** argv) {
// Initialize MPI environment
MPI_Init(&argc, &argv);
// Get the number of processes and the rank of this process
int num_procs, rank;
MPI_Comm_size(MPI_COMM_WORLD, &num_procs);
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
// Calculate the number of rows per process
int rows_per_proc = N / num_procs;
if (N % num_procs != 0 && rank == num_procs - 1) {
rows_per_proc += N % num_procs;
}
// Allocate memory for the local matrices
double* local_u = (double*) malloc((rows_per_proc + 2) * N * sizeof(double));
double* local_unew = (double*) malloc((rows_per_proc + 2) * N * sizeof(double));
// Initialize the local matrices
for (int i = 1; i <= rows_per_proc; i++) {
for (int j = 0; j < N; j++) {
if (i == 1 || i == rows_per_proc || j == 0 || j == N - 1) {
local_u[i * N + j] = 8.0;
local_unew[i * N + j] = 8.0;
} else {
local_u[i * N + j] = 0.0;
local_unew[i * N + j] = 0.0;
}
}
}
// Perform the Jacobi iteration
for (int iter = 0; iter < 10; iter++) {
// Exchange boundary rows with neighboring processes
if (rank > 0) {
MPI_Sendrecv(&local_u[N], N, MPI_DOUBLE, rank - 1, 0, &local_u[0], N, MPI_DOUBLE, rank - 1, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE);
}
if (rank < num_procs - 1) {
MPI_Sendrecv(&local_u[rows_per_proc * N], N, MPI_DOUBLE, rank + 1, 0, &local_u[(rows_per_proc + 1) * N], N, MPI_DOUBLE, rank + 1, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE);
}
// Perform the Jacobi update on the local matrix
for (int i = 2; i <= rows_per_proc + 1; i++) {
for (int j = 1; j < N - 1; j++) {
local_unew[i * N + j] = 0.25 * (local_u[(i - 1) * N + j] + local_u[(i + 1) * N + j] + local_u[i * N + j - 1] + local_u[i * N + j + 1]);
}
}
// Copy the updated local matrix to the original local matrix
for (int i = 1; i <= rows_per_proc; i++) {
for (int j = 0; j < N; j++) {
local_u[i * N + j] = local_unew[i * N + j];
}
}
}
// Gather the local matrices into the global matrix
double* u = NULL;
if (rank == 0) {
u = (double*) malloc(N * N * sizeof(double));
}
MPI_Gather(&local_u[N], rows_per_proc * N, MPI_DOUBLE, &u[N], rows_per_proc * N, MPI_DOUBLE, 0, MPI_COMM_WORLD);
// Print the global matrix
if (rank == 0) {
printf("Global matrix:\n");
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
printf("%f ", u[i * N + j]);
}
printf("\n");
}
}
// Free the memory
free(local_u);
free(local_unew);
if (rank == 0) {
free(u);
}
// Finalize MPI environment
MPI_Finalize();
return 0;
}
```
这个示例代码将一个16×16的矩阵分成若干块,并使用MPI在多个进程之间进行通信和计算。具体来说,每个进程都有一个局部矩阵,其中边缘元素被初始化为8,中间元素被初始化为0。在每次迭代中,进程之间通过MPI交换边缘元素,并使用Jacobi算法计算局部矩阵的更新。最后,每个进程将其局部矩阵的一部分收集到一个全局矩阵中,进程0将全局矩阵打印出来。
希望这个示例代码能够对您有所帮助!
相关推荐
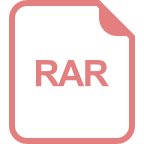











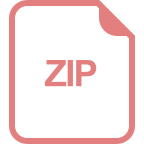
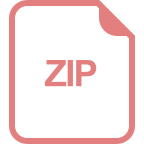