liunx中creat命令使用方法
时间: 2023-12-10 13:38:43 浏览: 90
`create` 是一个系统调用,用于创建一个新的文件。在 Linux 中,它的具体使用方法如下:
```
#include <fcntl.h>
int creat(const char *pathname, mode_t mode);
```
其中,`pathname` 是要创建的文件名,`mode` 是文件的权限。
如果文件成功创建,则返回文件描述符(file descriptor)。如果出现错误,则返回 `-1`。
使用示例:
```c
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
int main() {
int fd = creat("test.txt", 0644);
if (fd == -1) {
perror("creat");
exit(EXIT_FAILURE);
}
printf("Created file with file descriptor: %d\n", fd);
close(fd);
return 0;
}
```
这个例子创建了一个名为 `test.txt` 的文件,并将其权限设置为 `0644`。如果创建成功,它会输出创建的文件的文件描述符,然后关闭文件描述符。如果创建失败,它会输出一个错误信息并退出程序。
相关问题
在Linux中使用creat函数,创建目录LinuxPrj/Exp/temp.dat文件,
在Linux中,如果你想使用`creat()`函数来创建一个新的目录而不是文件,需要注意的是`creat()`是一个用于创建文件的系统调用,它并不适用于创建目录。如果你需要创建目录,应该使用`mkdir()`命令或者`filesystem_h`库中的`makedir()`函数。
如果你确实想通过程序操作创建目录,并且假设是在C语言环境中,你可以使用`mkdir()`函数,语法类似于:
```c
#include <sys/stat.h>
#include <unistd.h>
int mkdir(const char* path, mode_t mode);
```
例如,如果要在当前工作目录下创建名为"LinuxPrj/Exp/temp.dat"的目录,你可以这样做:
```c
char directory[] = "LinuxPrj/Exp/";
mode_t permissions = S_IRWXU | S_IRGRP | S_IXGRP | S_IROTH | S_IXOTH; // 指定权限
if (mkdir(directory, permissions) != 0) {
perror("Failed to create directory");
exit(EXIT_FAILURE);
} else {
printf("Directory created successfully.\n");
}
```
这里我们使用了`S_IRWXU`等位表示用户可以读、写和执行,`S_IRGRP`和`S_IXGRP`表示组成员有读取和执行权限,`S_IROTH`和`S_IXOTH`表示其他用户可以读取和执行。
linux写入文件命令
在Linux中,写入文件的常用命令是"write"和"echo"。
使用"write"命令可以将指定的内容写入到指定用户的终端。其语法为:
write [用户名 [终端 [文件名]
使用"echo"命令可以将指定的内容写入到指定的文件中。其语法为:
echo [内容 > [文件名]
另外,你还可以使用文件IO函数来在C语言中进行文件写入操作。例如,可以使用open函数打开文件,使用write函数将内容写入文件,最后使用close函数关闭文件。下面是一个示例代码:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
int main() {
int fd;
char *content = "file write";
fd = open("./file1", O_RDWR);
if (fd == -1) {
printf("open file1 failed\n");
fd = open("./file1", O_RDWR | O_CREAT, 0600);
if (fd > 0) {
printf("create file1 successed\n");
}
}
printf("open file1 successed, fd=%d\n", fd);
write(fd, content, strlen(content));
close(fd);
return 0;
}
请注意,在C语言中,使用文件IO函数进行文件写入操作时,需要包含相应的头文件,并在写入完成后关闭文件。
阅读全文
相关推荐
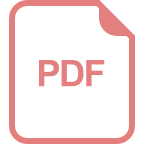
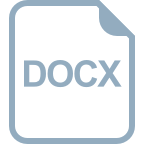
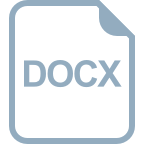













