C语言实现四元数单位化
时间: 2023-08-20 10:11:44 浏览: 53
四元数的单位化操作是将一个非零的四元数除以它的模长,使其成为单位四元数(模长为1)。C语言实现可以按照以下步骤进行:
1. 首先计算四元数的模长。对于一个四元数q = (w, x, y, z),模长为sqrt(w^2 + x^2 + y^2 + z^2)。
2. 如果四元数的模长为0,则该四元数无法进行单位化操作,直接返回原四元数。
3. 否则,将四元数的各个分量除以模长即可得到单位四元数。即q' = q / |q|,其中|q|表示q的模长。
下面是一个C语言实现的例子:
```c
#include <stdio.h>
#include <math.h>
typedef struct {
double w, x, y, z;
} Quaternion;
Quaternion normalize(Quaternion q) {
double norm = sqrt(q.w * q.w + q.x * q.x + q.y * q.y + q.z * q.z);
if (norm == 0) {
return q;
}
q.w /= norm;
q.x /= norm;
q.y /= norm;
q.z /= norm;
return q;
}
int main() {
Quaternion q = {2.0, 3.0, 4.0, 5.0};
q = normalize(q);
printf("Normalized quaternion: (%f, %f, %f, %f)\n", q.w, q.x, q.y, q.z);
return 0;
}
```
在上面的例子中,我们定义了一个Quaternion结构体来表示四元数,实现了一个normalize函数来对四元数进行单位化操作。在main函数中,我们定义了一个四元数q并对其进行单位化,最后输出结果。注意,在实际应用中可能需要考虑四元数的精度问题。
相关推荐
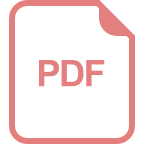
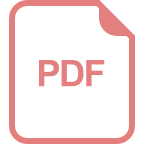














