arduino 舵机缓冲程序
时间: 2023-08-23 14:05:49 浏览: 80
以下是一个基本的 Arduino 舵机缓冲程序示例:
```
#include <Servo.h>
Servo myservo;
int pos = 0; // 定义舵机当前位置
int targetPos = 90; // 定义目标位置
int increment = 1; // 定义增量
void setup() {
myservo.attach(9); // 将舵机连接到引脚 9
}
void loop() {
if (pos != targetPos) { // 如果舵机未到达目标位置
if (pos < targetPos) { // 如果舵机当前位置比目标位置小
pos += increment; // 逐步增加位置
} else { // 如果舵机当前位置比目标位置大
pos -= increment; // 逐步减少位置
}
myservo.write(pos); // 将新位置传递给舵机
delay(15); // 等待一段时间,以使舵机移动到新位置
}
}
```
上述示例中,我们使用了 `Servo` 库来控制舵机。在 `setup` 函数中,我们将舵机连接到引脚 9。在 `loop` 函数中,我们使用一个 while 循环来逐步移动舵机到目标位置。我们使用 `delay` 函数来等待舵机到达新位置。在每次循环中,我们还可以更改目标位置,以模拟舵机移动的不同速度和轨迹。
相关问题
arduino舵机控制程序
以下是一个简单的 Arduino 舵机控制程序:
```c++
#include <Servo.h>
Servo myservo; // 创建舵机对象
int pos = 0; // 舵机位置变量
void setup() {
myservo.attach(9); // 将舵机连接到数字引脚 9
}
void loop() {
for (pos = 0; pos <= 180; pos += 1) { // 从 0 到 180 度逐步增加舵机位置
myservo.write(pos); // 将位置写入舵机
delay(15); // 等待舵机旋转到指定角度
}
for (pos = 180; pos >= 0; pos -= 1) { // 从 180 到 0 度逐步减小舵机位置
myservo.write(pos); // 将位置写入舵机
delay(15); // 等待舵机旋转到指定角度
}
}
```
这个程序使用了 Arduino 自带的 Servo 库,将一个舵机连接到数字引脚 9,并控制它从 0 到 180 度旋转,再从 180 到 0 度旋转,不断重复这个过程。你可以根据自己的需要修改舵机连接的引脚和舵机旋转的范围和速度。
arduino舵机程序
以下是一个基本的 Arduino 舵机程序示例:
```c++
#include <Servo.h>
Servo myservo; // 创建一个舵机对象
void setup() {
myservo.attach(9); // 将舵机连接到数字引脚 9
}
void loop() {
myservo.write(90); // 将舵机旋转到 90 度位置
delay(1000); // 等待 1 秒
myservo.write(0); // 将舵机旋转到 0 度位置
delay(1000); // 等待 1 秒
}
```
这个程序使用了 Servo 库,需要先在 Arduino IDE 中安装 Servo 库才能正常编译和上传。在 `setup()` 函数中,我们将舵机连接到数字引脚 9 上,然后在 `loop()` 函数中,我们先将舵机旋转到 90 度位置,等待 1 秒,然后将舵机旋转到 0 度位置,再等待 1 秒,然后重复这个过程。
阅读全文
相关推荐
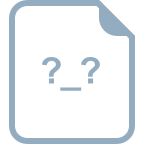
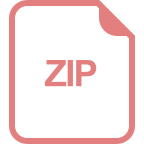













