优先队列式分支限界法01背包问题c++
时间: 2023-11-24 20:50:21 浏览: 30
以下是使用C++实现优先队列式分支限界法求解0-1背包问题的代码:
```c++
#include <iostream>
#include <queue>
#include <algorithm>
using namespace std;
struct Node {
int level; // 当前节点所在的层数
int profit; // 当前节点的价值
int weight; // 当前节点的重量
double bound; // 当前节点的价值上界
};
bool operator<(const Node& a, const Node& b) {
return a.bound < b.bound;
}
double bound(Node u, int n, int W, int* p, int* w) {
if (u.weight >= W) {
return 0;
}
double bound = u.profit;
int j = u.level + 1;
int totweight = u.weight;
while ((j < n) && (totweight + w[j] <= W)) {
totweight += w[j];
bound += p[j];
j++;
}
if (j < n) {
bound += (W - totweight) * p[j] / w[j];
}
return bound;
}
int knapsack(int n, int* p, int* w, int W) {
priority_queue<Node> Q;
Node u, v;
u.level = -1;
u.profit = 0;
u.weight = 0;
u.bound = bound(u, n, W, p, w);
int maxprofit = 0;
Q.push(u);
while (!Q.empty()) {
u = Q.top();
Q.pop();
if (u.bound > maxprofit) {
v.level = u.level + 1;
v.weight = u.weight + w[v.level];
v.profit = u.profit + p[v.level];
if (v.weight <= W && v.profit > maxprofit) {
maxprofit = v.profit;
}
v.bound = bound(v, n, W, p, w);
if (v.bound > maxprofit) {
Q.push(v);
}
v.weight = u.weight;
v.profit = u.profit;
v.bound = bound(v, n, W, p, w);
if (v.bound > maxprofit) {
Q.push(v);
}
}
}
return maxprofit;
}
int main() {
int n = 5;
int p[] = {3, 2, 4, 1, 5};
int w[] = {2, 1, 3, 2, 4};
int W = 7;
int maxprofit = knapsack(n, p, w, W);
cout << "Max profit: " << maxprofit << endl;
return 0;
}
```
相关推荐
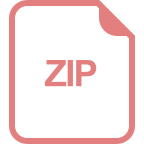















