class UNet3plus(tnn.Module) : """UNET3+ autoencoder for semantic segmentation.""" def __init__(self, n_classes = 2, in_channels = 3, depth = 3, first_output_channels = 8, upwards_feature_channels = 16, sideways_mask_shape = [200,200]): super().__init__() input_layer = ULayerDown(in_channels,first_output_channels) down_layers = [ULayerDown(first_output_channels*2**i,first_output_channels*2**(i+1)) for i in range(depth-1)] down_layers.append(ULayerDown(first_output_channels*2**(depth-1),upwards_feature_channels)) down_layers.insert(0,input_layer) self.seqdown = tnn.Sequential(*down_layers) up_layers = [] for i in range(depth) : up_layers.append(ULayerUp(upwards_feature_channels, n_classes, mask_shape = sideways_mask_shape, connected_nodes= down_layers[:depth-1-i]+ up_layers )) self.sequp = tnn.Sequential(*up_layers) self.classifier = ClassificationArm(upwards_feature_channels,n_classes) self.last_layer = tnn.Conv2d(upwards_feature_channels,n_classes,3,padding=1) self._side_mask_shape = sideways_mask_shape self.presence_prediction = None def forward(self,X): Y = tnn.functional.normalize(X,dim=1) Y = self.seqdown(Y) Y = tnn.Upsample(scale_factor=2)(Y) self.presence_prediction = self.classifier(Y) Y = self.sequp(Y) for layer in self.sequp : layer.side_mask_output *= self.presence_prediction.unsqueeze(-1).unsqueeze(-1) Y = self.last_layer(Y) Y*= self.presence_prediction.unsqueeze(-1).unsqueeze(-1) Y = tnn.Upsample(X.shape[-2:])(Y) return tnn.Softmax(dim=1)(Y)
时间: 2024-04-05 10:33:41 浏览: 118
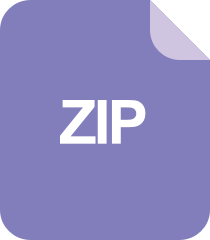
UNet3plus_pth:UNet3 + UNet ++ UNet,用于Pytorth的深度自动人像抠像中

这段代码是一个使用UNet3plus架构实现的语义分割的自动编码器。下面是对代码的解释:
- `UNet3plus`类继承自`tnn.Module`,用于构建UNET3自动编码器。
- 构造函数`__init__`接受一些参数,包括类别数(`n_classes`)、输入通道数(`in_channels`)、深度(`depth`)、第一个输出通道数(`first_output_channels`)、向上特征通道数(`upwards_feature_channels`)和侧面掩码形状(`sideways_mask_shape`)等。
- 构造函数中首先调用父类的构造函数`super().__init__()`来初始化父类的属性。
- 接下来创建输入层`input_layer`,并创建`depth`个下采样层`down_layers`,每个下采样层的输入通道数是前一层的两倍。
- 将输入层和下采样层添加到序列`seqdown`中。
- 接着创建`depth`个上采样层`up_layers`,每个上采样层的输入通道数是`upwards_feature_channels`,输出通道数是`n_classes`,并且指定侧面掩码形状和连接的下采样层。
- 将上采样层添加到序列`sequp`中。
- 创建分类器`classifier`,用于预测存在性特征。
- 创建最后一层卷积层`last_layer`,用于生成最终的分割结果。
- `_side_mask_shape`属性保存侧面掩码的形状。
- `presence_prediction`属性用于保存存在性特征的预测结果。
- `forward`方法实现了模型的前向传播过程。输入`X`经过归一化后,通过下采样层、上采样层和最后一层卷积层得到分割结果。同时,存在性特征的预测结果会乘以上采样层的侧面掩码输出,并将结果进行softmax归一化。
阅读全文
相关推荐
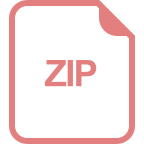
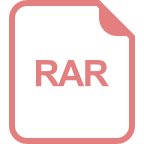















