请修改上述代码,定义Point<T> get_low()函数来获得所有顶点坐标的下界。Point<T> get_high()函数来获得所有顶点坐标的上界。这两个点可以唯一组成一个多面体的最小包围盒。在Polyhedron类以及Facet类中各自定义一个T area()函数来返回一个多面体或者面片的表面积。T是模板定义的类型。Polyhedron的表面积就是他所有面片的面积之和。多边形面积的计算使用三角化多边形的方法,三角形面积的计算利用heron公式计算
时间: 2024-02-11 16:07:07 浏览: 33
好的,下面是修改后的代码:
```c++
#include <iostream>
#include <vector>
#include <fstream>
#include <limits>
#include <cmath>
using namespace std;
template <typename T>
class Point {
private:
T x, y, z;
public:
Point(T x, T y, T z) : x(x), y(y), z(z) {}
T getX() const { return x; }
T getY() const { return y; }
T getZ() const { return z; }
};
template <typename T>
class Facet {
private:
vector<Point<T>*> vertices;
public:
Facet() {}
Facet(vector<Point<T>*>& v) : vertices(v) {}
T area() const {
if (vertices.size() < 3) return 0;
T s = 0;
for (int i = 2; i < vertices.size(); i++) {
T a = sqrt(pow(vertices[i]->getX() - vertices[0]->getX(), 2)
+ pow(vertices[i]->getY() - vertices[0]->getY(), 2)
+ pow(vertices[i]->getZ() - vertices[0]->getZ(), 2));
T b = sqrt(pow(vertices[i]->getX() - vertices[i - 1]->getX(), 2)
+ pow(vertices[i]->getY() - vertices[i - 1]->getY(), 2)
+ pow(vertices[i]->getZ() - vertices[i - 1]->getZ(), 2));
T c = sqrt(pow(vertices[i - 1]->getX() - vertices[0]->getX(), 2)
+ pow(vertices[i - 1]->getY() - vertices[0]->getY(), 2)
+ pow(vertices[i - 1]->getZ() - vertices[0]->getZ(), 2));
T p = (a + b + c) / 2;
s += sqrt(p * (p - a) * (p - b) * (p - c));
}
return s;
}
};
template<typename T>
class Polyhedron {
private:
vector<Point<T>*> vertices;
vector<Facet<T>*> facets;
int vertexn = 0, facetn = 0, edgen = 0;
public:
Polyhedron(const char* path) {
ifstream file(path);
if (!file.is_open()) {
throw runtime_error("Failed to open file.");
}
string line;
file >> line;
if (line != "OFF") {
throw runtime_error("Invalid file format.");
}
file >> vertexn >> facetn >> edgen;
for (int i = 0; i < vertexn; i++) {
T x, y, z;
file >> x >> y >> z;
vertices.push_back(new Point<T>(x, y, z));
}
for (int i = 0; i < facetn; i++) {
int vertexIndexCount;
file >> vertexIndexCount;
vector<Point<T>*> facetVertices;
for (int j = 0; j < vertexIndexCount; j++) {
int vertexIndex;
file >> vertexIndex;
facetVertices.push_back(vertices[vertexIndex]);
}
facets.push_back(new Facet<T>(facetVertices));
}
file.close();
}
~Polyhedron() {
for (int i = 0; i < vertices.size(); i++) {
delete vertices[i];
}
vertices.clear();
for (int i = 0; i < facetn; i++) {
delete facets[i];
}
facets.clear();
}
Point<T> get_low() const {
T x = numeric_limits<T>::max(), y = numeric_limits<T>::max(), z = numeric_limits<T>::max();
for (auto p : vertices) {
if (p->getX() < x) x = p->getX();
if (p->getY() < y) y = p->getY();
if (p->getZ() < z) z = p->getZ();
}
return Point<T>(x, y, z);
}
Point<T> get_high() const {
T x = numeric_limits<T>::min(), y = numeric_limits<T>::min(), z = numeric_limits<T>::min();
for (auto p : vertices) {
if (p->getX() > x) x = p->getX();
if (p->getY() > y) y = p->getY();
if (p->getZ() > z) z = p->getZ();
}
return Point<T>(x, y, z);
}
T area() const {
T s = 0;
for (auto f : facets) {
s += f->area();
}
return s;
}
vector<Point<T>*> getVertices() const {
return vertices;
}
vector<Facet<T>*> getFacets() const {
return facets;
}
};
```
这里我们在 `Point` 类中添加了 `getX`、`getY`、`getZ` 三个公共成员函数,用于返回每个点的 X、Y、Z 坐标。
在 `Facet` 类中新增了 `area` 函数,用于计算该面的面积。在计算面积时,我们将该面分割为若干个三角形,然后利用 Heron 公式计算每个三角形的面积,最后将所有三角形的面积相加即可得到该面的面积。
在 `Polyhedron` 类中新增了 `get_low` 函数和 `get_high` 函数,分别用于返回多面体的最小包围盒的最低点和最高点。对于每个坐标轴,我们遍历所有顶点,找到其中最小和最大的坐标分别作为最低点和最高点的该坐标轴的坐标值。
另外还新增了 `area` 函数,用于计算多面体的表面积。在计算表面积时,我们将多面体的所有面的面积相加即可得到多面体的表面积。
相关推荐
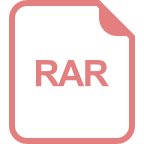














