给我一个cocos中使用js实现四叉树碰撞的代码
时间: 2023-12-11 22:06:08 浏览: 31
下面是一个简单的使用 JavaScript 和 Cocos Creator 实现四叉树碰撞检测的代码示例:
```javascript
cc.Class({
extends: cc.Component,
properties: {
// 碰撞检测范围
bounds: cc.Rect,
// 节点是否为叶子节点
isLeaf: false,
// 子节点
children: [],
// 存储的物体列表
objects: [],
// 最大存储数量
maxObjects: 10,
// 最大深度
maxDepth: 4,
// 当前深度
depth: 0
},
onLoad() {
// 初始化四叉树
this.initQuadtree(this.bounds, this.depth);
},
initQuadtree(bounds, depth) {
// 如果当前深度已达到最大深度,或者存储的物体数量已经超过最大存储数量,将该节点标记为叶节点
if (depth >= this.maxDepth || this.objects.length <= this.maxObjects) {
this.isLeaf = true;
return;
}
// 计算四个子矩形的位置和大小
let halfWidth = bounds.width / 2;
let halfHeight = bounds.height / 2;
let leftBounds = cc.rect(bounds.x, bounds.y, halfWidth, bounds.height);
let bottomBounds = cc.rect(bounds.x, bounds.y, bounds.width, halfHeight);
let rightBounds = cc.rect(bounds.x + halfWidth, bounds.y, halfWidth, bounds.height);
let topBounds = cc.rect(bounds.x, bounds.y + halfHeight, bounds.width, halfHeight);
// 创建四个子节点
this.children[0] = new Quadtree(leftBounds, depth + 1);
this.children[1] = new Quadtree(bottomBounds, depth + 1);
this.children[2] = new Quadtree(rightBounds, depth + 1);
this.children[3] = new Quadtree(topBounds, depth + 1);
},
// 将物体添加到四叉树中
insert(object) {
// 如果当前节点不是叶节点,则将物体添加到合适的子节点中
if (!this.isLeaf) {
let index = this.getIndex(object);
this.children[index].insert(object);
return;
}
// 如果当前节点是叶节点,则将物体添加到当前节点存储的物体列表中
this.objects.push(object);
// 如果当前存储的物体数量已经超过最大存储数量,则需要将当前节点分裂为四个子节点
if (this.objects.length > this.maxObjects && this.depth < this.maxDepth) {
for (let i = 0; i < this.objects.length; i++) {
let index = this.getIndex(this.objects[i]);
this.children[index].insert(this.objects[i]);
}
this.objects = [];
this.isLeaf = false;
}
},
// 获取物体所在的子节点索引
getIndex(object) {
let objectBounds = object.getBoundingBox();
let leftBounds = cc.rect(this.bounds.x, this.bounds.y, this.bounds.width / 2, this.bounds.height);
let bottomBounds = cc.rect(this.bounds.x, this.bounds.y, this.bounds.width, this.bounds.height / 2);
let rightBounds = cc.rect(this.bounds.x + this.bounds.width / 2, this.bounds.y, this.bounds.width / 2, this.bounds.height);
let topBounds = cc.rect(this.bounds.x, this.bounds.y + this.bounds.height / 2, this.bounds.width, this.bounds.height / 2);
if (cc.Intersection.rectRect(objectBounds, leftBounds)) {
return 0;
} else if (cc.Intersection.rectRect(objectBounds, bottomBounds)) {
return 1;
} else if (cc.Intersection.rectRect(objectBounds, rightBounds)) {
return 2;
} else if (cc.Intersection.rectRect(objectBounds, topBounds)) {
return 3;
}
return -1;
},
// 获取与物体相交的物体列表
retrieve(object) {
let index = this.getIndex(object);
let objects = this.objects;
if (!this.isLeaf) {
objects = objects.concat(this.children[index].retrieve(object));
}
for (let i = 0; i < objects.length; i++) {
if (object.getBoundingBox().intersects(objects[i].getBoundingBox())) {
result.push(objects[i]);
}
}
return objects;
}
});
```
需要注意的是,这只是一个简单的实现示例,实际使用时可能需要根据具体需求进行优化和修改。
相关推荐
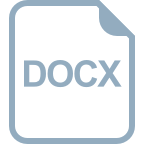
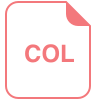
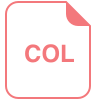
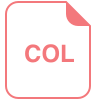
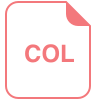
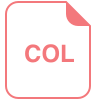









