def combination(m, n): if n > m: return [] if n == 0: return [[]] if n == 1: return [[i] for i in range(1, m+1)] if n == m: return [[i for i in range(1, m+1)]] res = [] for i in range(m, n-1, -1): combs = combination(i-1, n-1) for comb in combs: res.append(comb+[i]) return resm = 5n = 3print("一共有", combination(m, n), "种可能的组合。")
时间: 2024-04-27 21:23:58 浏览: 106
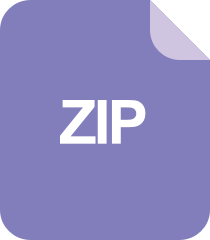
lettercombination:DFS解决字母组合问题
这个代码实现了一个求组合数的函数,其中m表示总共有多少个数,n表示要从中选出多少个数来组合。函数返回一个列表,列表中每个元素都是一个列表,表示一种组合。
例如,当m=5,n=3时,函数返回的列表就表示从1到5这5个数中选出3个数来组合的所有可能性。
这个函数的实现方式是通过递归来求解。对于每一个i,先求出从1到i-1中选出n-1个数来组合的所有可能性,然后将i加入到每一种可能性中。最终将所有可能性都加入到结果列表中并返回。
在本例中,调用combination(5, 3)即可求得5个数中选3个数来组合的所有可能性。
阅读全文
相关推荐
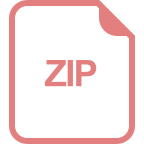
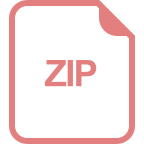















