C语言数据结构写代码:编写一个表达式中括号是否合法配对的算法。A:开闭括号B:多种括号。
时间: 2024-12-06 21:22:21 浏览: 14
在C语言中,我们可以使用栈(Stack)数据结构来检查表达式中括号的配对是否合法。这里我们假设只处理最简单的"()"和"[]"两种括号。以下是基本的算法步骤:
```c
#include <stdbool.h>
#include <stdio.h>
typedef enum { OPEN_PAREN, CLOSE_PAREN } BracketType;
const size_t MAX_SIZE = 100; // 栈的最大容量
bool isValidExpression(char* expression) {
stacksize_t count[MAX_SIZE] = {0}; // 初始化一个大小为MAX_SIZE的栈
BracketType currentBracket = OPEN_PAREN;
for (int i = 0; expression[i]; i++) {
char ch = expression[i];
if (ch == '(' || ch == '[') {
count[currentBracket]++;
currentBracket = ch == '(' ? OPEN_PAREN : CLOSE_PAREN;
} else if (ch == ')' || ch == ']') {
if (count[currentBracket] > 0) {
count[currentBracket]--;
if (!count[currentBracket]) {
currentBracket = OPEN_PAREN; // 如果当前栈顶元素匹配当前关闭的括号,清空栈并继续
}
} else {
return false; // 遇到未匹配的关闭括号,直接返回false
}
} else {
continue; // 非括号字符跳过
}
if (i == strlen(expression) - 1 && count[CLOSE_PAREN] != 0) {
return false; // 结束遍历但栈内还有剩余的关闭括号,说明不匹配
}
}
return count[CLOSE_PAREN] == 0; // 检查栈是否为空,表示所有括号都已配对
}
int main() {
char expr[] = "((()))";
if (isValidExpression(expr)) {
printf("The expression is valid.\n");
} else {
printf("The expression is not valid.\n");
}
return 0;
}
```
阅读全文
相关推荐
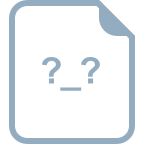
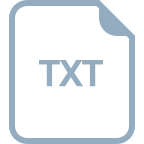















