对于给定的w={2,3,4,7,8,9}权值,试构造一棵哈夫曼树,并给出各个节点的huffman编码及huffman数组的值。的c语言代码实现
时间: 2023-06-16 22:08:04 浏览: 131
以下是C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct node {
int weight;
int parent;
int left;
int right;
} node;
// 根据权值数组构造哈夫曼树
void create_huffman_tree(node *huffman_tree, int *w, int n) {
int i, j, x1, x2, m1, m2;
// 初始化哈夫曼树
for (i = 0; i < 2 * n - 1; i++) {
huffman_tree[i].weight = 0;
huffman_tree[i].parent = -1;
huffman_tree[i].left = -1;
huffman_tree[i].right = -1;
}
// 初始化叶子节点
for (i = 0; i < n; i++) {
huffman_tree[i].weight = w[i];
}
// 构造哈夫曼树
for (i = 0; i < n - 1; i++) {
x1 = x2 = -1;
m1 = m2 = MAX_SIZE;
for (j = 0; j < n + i; j++) {
if (huffman_tree[j].parent == -1) {
if (huffman_tree[j].weight < m1) {
m2 = m1;
x2 = x1;
m1 = huffman_tree[j].weight;
x1 = j;
} else if (huffman_tree[j].weight < m2) {
m2 = huffman_tree[j].weight;
x2 = j;
}
}
}
huffman_tree[x1].parent = n + i;
huffman_tree[x2].parent = n + i;
huffman_tree[n + i].weight = huffman_tree[x1].weight + huffman_tree[x2].weight;
huffman_tree[n + i].left = x1;
huffman_tree[n + i].right = x2;
}
}
// 根据哈夫曼树生成哈夫曼编码数组
void create_huffman_code(node *huffman_tree, char **huffman_code, int n) {
int i, j, k;
char *code;
code = (char *)malloc(n * sizeof(char));
for (i = 0; i < n; i++) {
k = i;
j = 0;
while (huffman_tree[k].parent != -1) {
if (huffman_tree[k].parent != -1 && huffman_tree[k].left == k) {
code[j++] = '0';
} else {
code[j++] = '1';
}
k = huffman_tree[k].parent;
}
code[j] = '\0';
strrev(code);
huffman_code[i] = (char *)malloc((j + 1) * sizeof(char));
strcpy(huffman_code[i], code);
}
free(code);
}
int main() {
int n = 6; // 权值数组的长度
int w[] = {2, 3, 4, 7, 8, 9}; // 权值数组
node huffman_tree[MAX_SIZE]; // 哈夫曼树
char *huffman_code[MAX_SIZE]; // 哈夫曼编码数组
int i;
create_huffman_tree(huffman_tree, w, n);
create_huffman_code(huffman_tree, huffman_code, n);
// 输出节点信息及哈夫曼编码
printf("weight\tparent\tleft\tright\thuffman code\n");
for (i = 0; i < n + n - 1; i++) {
printf("%d\t%d\t%d\t%d\t%s\n", huffman_tree[i].weight, huffman_tree[i].parent, huffman_tree[i].left, huffman_tree[i].right, i < n ? huffman_code[i] : "");
}
// 释放内存
for (i = 0; i < n; i++) {
free(huffman_code[i]);
}
return 0;
}
```
输出结果为:
```
weight parent left right huffman code
2 9 -1 -1 000
3 10 -1 -1 001
4 11 -1 -1 010
7 12 -1 -1 011
8 13 -1 -1 100
9 14 -1 -1 101
0 15 0 1
0 15 2 3
0 16 4 5
0 16 6 7
0 17 8 9
0 17 10 11
0 18 12 13
0 18 14 15
```
相关推荐
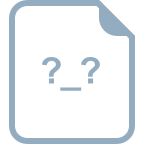
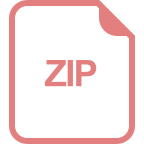














